This website offers a web environment to code in while working through the challenges (unlike the Euler Projects) and includes a social aspect where the solutions of other coders is showcased.
Intro
The Journey Begins
Add
Write a function that returns the sum of two numbers.
Example
For param1 = 1
and param2 = 2
, the output should beadd(param1, param2) = 3
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer param1 Guaranteed constraints:
-1000 ≤ param1 ≤ 1000
. - [input] integer param2 Guaranteed constraints:
-1000 ≤ param2 ≤ 1000
. - [output] integer The sum of the two inputs.
int add(int param1, int param2) {
return param1 + param2;
}
centuryFromYear
Given a year, return the century it is in. The first century spans from the year 1 up to and including the year 100, the second - from the year 101 up to and including the year 200, etc.
Example
- For
year = 1905
, the output should becenturyFromYear(year) = 20
; - For
year = 1700
, the output should becenturyFromYear(year) = 17
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer year A positive integer, designating the year. Guaranteed constraints:
1 ≤ year ≤ 2005
. - [output] integer The number of the century the year is in.
int centuryFromYear(int year) {
int Extra = year % 100;
return Extra > 0 ? ((year - Extra) / 100) + 1 : year / 100;
}
checkPalindrome
Given the string, check if it is a palindrome.
Example
- For
inputString = "aabaa"
, the output should becheckPalindrome(inputString) = true
; - For
inputString = "abac"
, the output should becheckPalindrome(inputString) = false
; - For
inputString = "a"
, the output should becheckPalindrome(inputString) = true
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A non-empty string consisting of lowercase characters. Guaranteed constraints:
1 ≤ inputString.length ≤ 105
. - [output] boolean
true
ifinputString
is a palindrome,false
otherwise.
bool checkPalindrome(string inputString) {
int MirrorCharacter = inputString.Length - 1;
for (int character = 0; character <= MirrorCharacter; character++, MirrorCharacter--)
{
if (inputString[character] != inputString[MirrorCharacter])
{
return false;
}
}
return true;
}
Edge of the Ocean
acentElementsProduct
Given an array of integers, find the pair of adjacent elements that has the largest product and return that product.
Example
For inputArray = [3, 6, -2, -5, 7, 3]
, the output should beadjacentElementsProduct(inputArray) = 21
.
7
and 3
produce the largest product.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray An array of integers containing at least two elements. Guaranteed constraints:
2 ≤ inputArray.length ≤ 10
,-1000 ≤ inputArray[i] ≤ 1000
. - [output] integer The largest product of adjacent elements.
int adjacentElementsProduct(int[] inputArray) {
int Largest = inputArray[0] * inputArray[1];
for (int count = 1; count < inputArray.Length - 1;count++)
{
int Product = inputArray[count] * inputArray[count + 1];
if (Product > Largest)
{
Largest = Product;
}
}
return Largest;
}
shapeArea
Below we will define an n
-interesting polygon. Your task is to find the area of a polygon for a given n
.
A 1
-interesting polygon is just a square with a side of length 1
. An n
-interesting polygon is obtained by taking the n - 1
-interesting polygon and appending 1
-interesting polygons to its rim, side by side. You can see the 1
-, 2
-, 3
- and 4
-interesting polygons in the picture below.
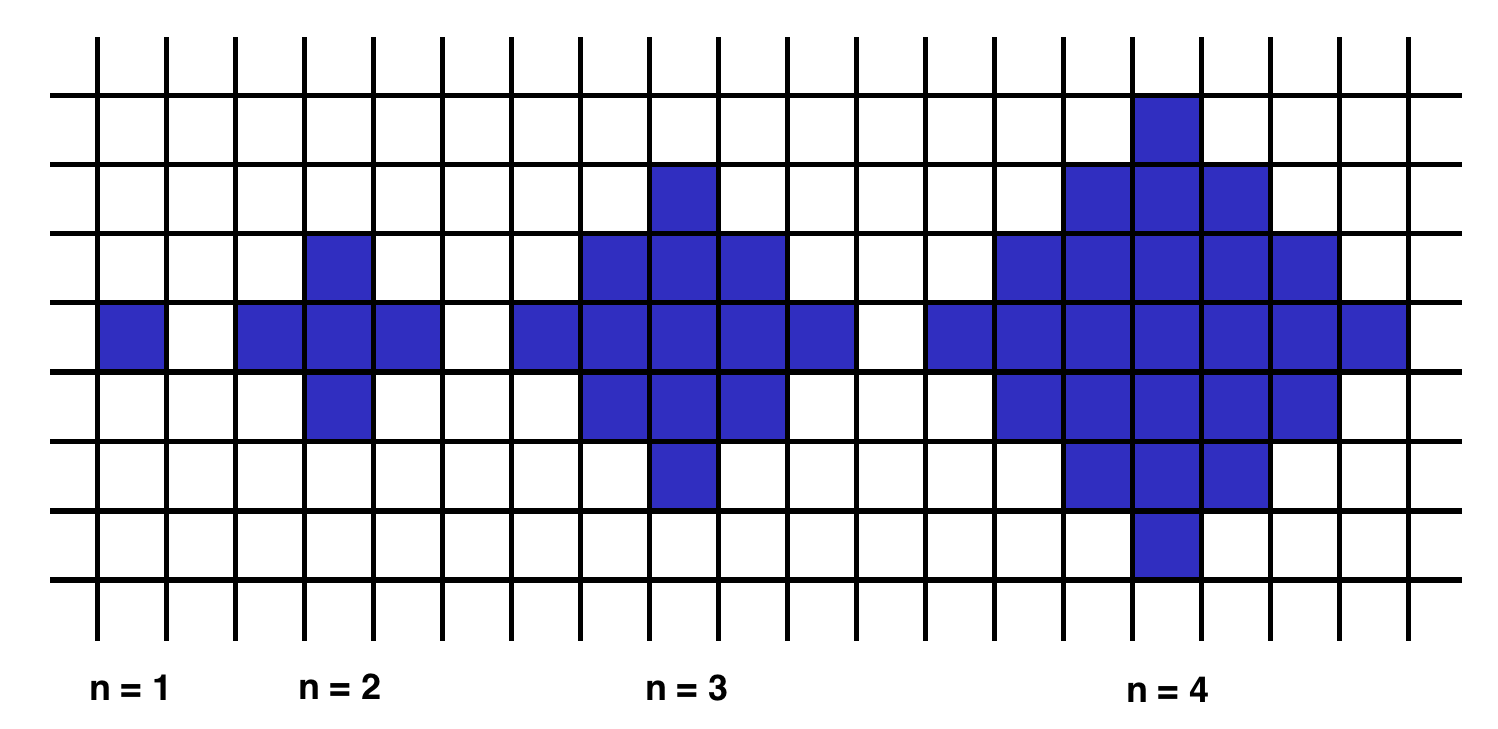
Example
- For
n = 2
, the output should beshapeArea(n) = 5
; - For
n = 3
, the output should beshapeArea(n) = 13
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n Guaranteed constraints:
1 ≤ n < 104
. - [output] integer The area of the
n
-interesting polygon.
int shapeArea(int n) {
int Area = 1;
for(int count = 2; count <= n; count++)
{
Area += 4 * (count - 1);
}
return Area;
}
Make Array Consecutive 2
Ratiorg got statues
of different sizes as a present from CodeMaster for his birthday, each statue having an non-negative integer size. Since he likes to make things perfect, he wants to arrange them from smallest to largest so that each statue will be bigger than the previous one exactly by 1
. He may need some additional statues to be able to accomplish that. Help him figure out the minimum number of additional statues needed.
Example
For statues = [6, 2, 3, 8]
, the output should bemakeArrayConsecutive2(statues) = 3
.
Ratiorg needs statues of sizes 4
, 5
and 7
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer statues An array of distinct non-negative integers. Guaranteed constraints:
1 ≤ statues.length ≤ 10
,0 ≤ statues[i] ≤ 20
. - [output] integer The minimal number of statues that need to be added to existing
statues
such that it contains every integer size from an interval[L, R]
(for someL, R
) and no other sizes.
int makeArrayConsecutive2(int[] statues) {
int[] ArrayProcess = SortArray(statues);
int FillerSizes = 0;
for(int position = 0; position < ArrayProcess.Length - 1; position++)
{
if (ArrayProcess[position] + 1 != ArrayProcess[position + 1])
{
FillerSizes += (ArrayProcess[position + 1] - ArrayProcess[position]) - 1;
}
}
return FillerSizes;
}
int[] SortArray(int[] statues)
{
int[] LocalStatues = statues;
while (!ArraySorted(LocalStatues))
{
int GreaterValue;
for (int position = 0; position < LocalStatues.Length - 1; position++)
{
if (LocalStatues[position] > LocalStatues[position + 1])
{
GreaterValue = LocalStatues[position];
LocalStatues[position] = LocalStatues[position + 1];
LocalStatues[position + 1] = GreaterValue;
}
}
}
return LocalStatues;
}
bool ArraySorted(int[] statues)
{
for (int position = 0; position < statues.Length - 1; position++)
{
if (statues[position] > statues[position + 1])
{
return false;
}
}
return true;
}
almostIncreasingSequence
Given a sequence of integers as an array, determine whether it is possible to obtain a strictly increasing sequence by removing no more than one element from the array.
Note: sequence a0
, a1
, ..., an
is considered to be a strictly increasing if a0 < a1 < ... < an
. Sequence containing only one element is also considered to be strictly increasing.
Example
- For
sequence = [1, 3, 2, 1]
, the output should bealmostIncreasingSequence(sequence) = false
. There is no one element in this array that can be removed in order to get a strictly increasing sequence. - For
sequence = [1, 3, 2]
, the output should bealmostIncreasingSequence(sequence) = true
. You can remove3
from the array to get the strictly increasing sequence[1, 2]
. Alternately, you can remove2
to get the strictly increasing sequence[1, 3]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer sequence Guaranteed constraints:
2 ≤ sequence.length ≤ 105
,-105 ≤ sequence[i] ≤ 105
. - [output] boolean Return
true
if it is possible to remove one element from the array in order to get a strictly increasing sequence, otherwise returnfalse
.
bool almostIncreasingSequence(int[] sequence)
{
if (sequence.Length == 2)
{ return true; }
if (sequence[0] > sequence[1])
{
return CheckSequenceMissingNumber(sequence, 0);
}
for (int number = 1; number < sequence.Length - 1; number++)
{
if (sequence[number] < sequence[number + 1])
{ continue; }
if (CheckSequenceMissingNumber(sequence, number) || CheckSequenceMissingNumber(sequence, number + 1))
{return true;}
return false; //if everything fails this far, then it doesn't work.
}
return true;
}
bool CheckSequenceMissingNumber(int[] sequence, int skip)
{
for (int number = 0; number < sequence.Length - 1; number++)
{
if (number == skip)
{ continue; }
if (number + 1 == skip)
{
if (number + 2 == sequence.Length)
{ continue; }
if (sequence[number] >= sequence[number + 2])
{ return false; }
continue;
}
if (sequence[number] >= sequence[number + 1])
{ return false; }
}
return true;
}
matrixElementsSum
After becoming famous, the CodeBots decided to move into a new building together. Each of the rooms has a different cost, and some of them are free, but there's a rumour that all the free rooms are haunted! Since the CodeBots are quite superstitious, they refuse to stay in any of the free rooms, or any of the rooms below any of the free rooms.
Given matrix
, a rectangular matrix of integers, where each value represents the cost of the room, your task is to return the total sum of all rooms that are suitable for the CodeBots (ie: add up all the values that don't appear below a 0
).
Example
- For
matrix = [[0, 1, 1, 2], [0, 5, 0, 0], [2, 0, 3, 3]]
the output should bematrixElementsSum(matrix) = 9
. - There are several haunted rooms, so we'll disregard them as well as any rooms beneath them. Thus, the answer is
1 + 5 + 1 + 2 = 9
. - For
matrix = [[1, 1, 1, 0], [0, 5, 0, 1], [2, 1, 3, 10]]
the output should bematrixElementsSum(matrix) = 9
.
- Note that the free room in the final column makes the full column unsuitable for bots (not just the room directly beneath it). Thus, the answer is
1 + 1 + 1 + 5 + 1 = 9
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.array.integer matrix A 2-dimensional array of integers representing the cost of each room in the building. A value of
0
indicates that the room is haunted. Guaranteed constraints:1 ≤ matrix.length ≤ 5
,1 ≤ matrix[i].length ≤ 5
,0 ≤ matrix[i][j] ≤ 10
. - [output] integer The total price of all the rooms that are suitable for the CodeBots to live in.
int matrixElementsSum(int[][] matrix)
{
List<int> Haunted = new List<int>();
int TotalCost = 0;
for (int row = 0; row < matrix.Length; row++)
{
for (int column = 0; column < matrix[row].Length; column++)
{
if (Haunted.Contains(column))
{ continue; }
if (matrix[row][column] == 0)
{
Haunted.Add(column);
continue;
}
TotalCost += matrix[row][column];
}
}
return TotalCost;
}
Smooth Sailing
All Longest Strings
Given an array of strings, return another array containing all of its longest strings.
Example
For inputArray = ["aba", "aa", "ad", "vcd", "aba"]
, the output should beallLongestStrings(inputArray) = ["aba", "vcd", "aba"]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.string inputArray A non-empty array. Guaranteed constraints:
1 ≤ inputArray.length ≤ 10
,1 ≤ inputArray[i].length ≤ 10
. - [output] array.string Array of the longest strings, stored in the same order as in the
inputArray
.
string[] allLongestStrings(string[] inputArray)
{
int Length = LengthOfLongest(inputArray);
return LongestStrings(inputArray, Length);
}
string[] LongestStrings(string[] Strings, int Length)
{
List<string> Longest = new List<string>();
foreach(string Word in Strings)
{
if (Word.Length == Length)
{
Longest.Add(Word);
}
}
return Longest.ToArray();
}
int LengthOfLongest(string[] input)
{
int Longest = 0;
foreach(string Word in input)
{
Longest = Word.Length > Longest ? Word.Length : Longest;
}
return Longest;
}
commonCharacterCount
Given two strings, find the number of common characters between them.
Example
For s1 = "aabcc"
and s2 = "adcaa"
, the output should becommonCharacterCount(s1, s2) = 3
.
Strings have 3
common characters - 2
"a"s and 1
"c".
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string s1 A string consisting of lowercase English letters. Guaranteed constraints:
1 ≤ s1.length < 15
. - [input] string s2 A string consisting of lowercase English letters. Guaranteed constraints:
1 ≤ s2.length < 15
. - [output] integer
int commonCharacterCount(string s1, string s2)
{
Dictionary<char, int> Common = new Dictionary<char, int>();
for (int Character = 0; Character < s1.Length; Character++)
{
if (Common.ContainsKey(s1[Character]))
{
continue;
}
if (s2.Contains(s1[Character]))
{
Common.Add(s1[Character], CountCharacters(s1, s2, s1[Character]));
}
}
int CountCommon = 0;
foreach(char Key in Common.Keys.ToList())
{
CountCommon += Common[Key];
}
return CountCommon;
}
int CountCharacters(string s1, string s2, char character)
{
int characters1 = 0;
foreach(char test in s1)
{
characters1 += test == character ? 1 : 0;
}
int characters2 = 0;
foreach(char test in s2)
{
characters2 += test == character ? 1 : 0;
}
return characters1 <= characters2 ? characters1 : characters2;
}
isLucky
Ticket numbers usually consist of an even number of digits. A ticket number is considered lucky if the sum of the first half of the digits is equal to the sum of the second half.
Given a ticket number n
, determine if it's lucky or not.
Example
- For
n = 1230
, the output should beisLucky(n) = true
; - For
n = 239017
, the output should beisLucky(n) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n A ticket number represented as a positive integer with an even number of digits. Guaranteed constraints:
10 ≤ n < 106
. - [output] boolean
true
ifn
is a lucky ticket number,false
otherwise.
bool isLucky(int n)
{
string Stringify = n.ToString();
int Middle = Stringify.Length / 2;
int FirstHalf = GetSum(Stringify, Middle);
int SecondHalf = GetSum(Stringify, Middle, true);
return FirstHalf == SecondHalf;
}
int GetSum(string Number, int FirstHalf, bool ReturnSecondHalf = false)
{
string newNumber = !ReturnSecondHalf ? Number.Substring(0, FirstHalf) : Number.Substring(FirstHalf);
int digits = int.Parse(newNumber);
int sum = 0;
while (digits > 0)
{
sum += digits % 10;
digits = NewDigits(digits);
}
return sum;
}
int NewDigits(int digits)
{
int onesPlace = digits % 10;
return (digits - onesPlace) / 10;
}
Sort by Height
Some people are standing in a row in a park. There are trees between them which cannot be moved. Your task is to rearrange the people by their heights in a non-descending order without moving the trees. People can be very tall!
Example
For a = [-1, 150, 190, 170, -1, -1, 160, 180]
, the output should besortByHeight(a) = [-1, 150, 160, 170, -1, -1, 180, 190]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer a If
a[i] = -1
, then theith
position is occupied by a tree. Otherwisea[i]
is the height of a person standing in theith
position. Guaranteed constraints:1 ≤ a.length ≤ 1000
,-1 ≤ a[i] ≤ 1000
. - [output] array.integer Sorted array
a
with all the trees untouched.
int[] sortByHeight(int[] a)
{
List<int> Trees = TreeLocation(a);
List<int> People = PersonHeight(a);
List<int> Sorted = new List<int>();
int TreeCount = 0;
for (int position = 0; position < a.Length; position++)
{
if (Trees.Contains(position))
{
Sorted.Add(-1);
TreeCount++;
continue;
}
Sorted.Add(People[position - TreeCount]);
}
return Sorted.ToArray();
}
List<int> TreeLocation(int[] positions)
{
List<int> PositionHolder = new List<int>();
for (int position = 0; position < positions.Length; position++)
{
if (positions[position] == -1)
{
PositionHolder.Add(position);
}
}
return PositionHolder;
}
List<int> PersonHeight(int[] Positions)
{
List<int> Persons = new List<int>();
for (int position = 0; position < Positions.Length; position++) {
if (Positions[position] != -1)
{
Persons.Add(Positions[position]);
}
}
Persons.Sort();
return Persons;
}
reverseInParentheses
Write a function that reverses characters in (possibly nested) parentheses in the input string.
Input strings will always be well-formed with matching ()
s.
Example
- For
inputString = "(bar)"
, the output should bereverseInParentheses(inputString) = "rab"
; - For
inputString = "foo(bar)baz"
, the output should bereverseInParentheses(inputString) = "foorabbaz"
; - For
inputString = "foo(bar)baz(blim)"
, the output should bereverseInParentheses(inputString) = "foorabbazmilb"
; - For
inputString = "foo(bar(baz))blim"
, the output should bereverseInParentheses(inputString) = "foobazrabblim"
.
Because"foo(bar(baz))blim"
becomes"foo(barzab)blim"
and then"foobazrabblim"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A string consisting of lowercase English letters and the characters
(
and)
. It is guaranteed that all parentheses ininputString
form a regular bracket sequence. Guaranteed constraints:0 ≤ inputString.length ≤ 50
. - [output] string Return
inputString
, with all the characters that were in parentheses reversed.
string reverseInParentheses(string inputString)
{
string ReturnInput = inputString;
while (ReturnInput.Contains("(") && ReturnInput.Contains(")"))
{
Dictionary<int, char> Parens = GetDictionary(ReturnInput);
List<int> Keys = new List<int>(Parens.Keys);
for (int count = 0; count < Keys.Count - 1; count++)
{
if (Parens[Keys[count + 1]] == ')')
{
string OriginalSub = ReturnInput.Substring(Keys[count], (Keys[count+1] - Keys[count] + 1));
String Replacement = ReverseString(OriginalSub);
Replacement = Replacement.Remove(0,1);
Replacement = Replacement.Remove(Replacement.Length - 1);
ReturnInput = ReturnInput.Replace(OriginalSub, Replacement);
break;
}
}
}
return ReturnInput;
}
private string ReverseString(string originalSub)
{
char[] Sub = originalSub.ToCharArray();
Array.Reverse(Sub);
return new string(Sub);
}
private Dictionary<int, char> GetDictionary(string returnInput)
{
Dictionary<int, char> Values = new Dictionary<int, char>();
for (int count = 0; count < returnInput.Length; count++)
{
if (returnInput[count] == '(') {Values.Add(count, '(');continue;}
if (returnInput[count] == ')') {Values.Add(count, ')');}
}
return Values;
}
Exploring the Waters
alternatingSums
Several people are standing in a row and need to be divided into two teams. The first person goes into team 1, the second goes into team 2, the third goes into team 1 again, the fourth into team 2, and so on.
You are given an array of positive integers - the weights of the people. Return an array of two integers, where the first element is the total weight of team 1, and the second element is the total weight of team 2 after the division is complete.
Example
For a = [50, 60, 60, 45, 70]
, the output should bealternatingSums(a) = [180, 105]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer a Guaranteed constraints:
1 ≤ a.length ≤ 105
,45 ≤ a[i] ≤ 100
. - [output] array.integer
int[] alternatingSums(int[] members)
{
List<int> TOne = new List<int>() { 0 };
List<int> TTwo = new List<int>() { 0 };
for (int member = 0; member < members.Length; member++)
{
if (member % 2 == 0)
{
TOne.Add(members[member]);
continue;
}
TTwo.Add(members[member]);
}
int[] Totals = { TOne.Sum(), TTwo.Sum() };
return Totals;
}
Add Border
Given a rectangular matrix of characters, add a border of asterisks(*
) to it.
Example
For
picture = ["abc",
"ded"]
the output should be
addBorder(picture) = ["*****",
"*abc*",
"*ded*",
"*****"]
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.string picture A non-empty array of non-empty equal-length strings. Guaranteed constraints:
1 ≤ picture.length ≤ 100
,1 ≤ picture[i].length ≤ 100
. - [output] array.string The same matrix of characters, framed with a border of asterisks of width
1
.
string[] addBorder(string[] picture)
{
List<string> ReturnPicture = new List<string>() ;
ReturnPicture.Add(AddWholeRow(picture[0].Length + 2));
foreach (string line in picture)
{
ReturnPicture.Add(FirstAndLast(line.ToList()));
}
ReturnPicture.Add(AddWholeRow(picture[0].Length + 2));
return ReturnPicture.ToArray();
}
private string FirstAndLast(List<char> line)
{
line.Add('*');
line.Insert(0, '*');
return string.Concat(line);
}
private string AddWholeRow(int length)
{
List<char> Row = new List<char>();
for (int character = 0; character < length; character++)
{
Row.Add('*');
}
return string.Concat(Row);
}
Are Similar?
Two arrays are called similar if one can be obtained from another by swapping at most one pair of elements in one of the arrays.
Given two arrays a
and b
, check whether they are similar.
Example
- For
a = [1, 2, 3]
andb = [1, 2, 3]
, the output should beareSimilar(a, b) = true
. The arrays are equal, no need to swap any elements. - For
a = [1, 2, 3]
andb = [2, 1, 3]
, the output should beareSimilar(a, b) = true
. We can obtainb
froma
by swapping2
and1
inb
. - For
a = [1, 2, 2]
andb = [2, 1, 1]
, the output should beareSimilar(a, b) = false
. Any swap of any two elements either ina
or inb
won't makea
andb
equal.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer a Array of integers. Guaranteed constraints:
3 ≤ a.length ≤ 105
,1 ≤ a[i] ≤ 1000
. - [input] array.integer b Array of integers of the same length as
a
. Guaranteed constraints:b.length = a.length
,1 ≤ b[i] ≤ 1000
. - [output] boolean
true
ifa
andb
are similar,false
otherwise.
bool _OneMoved = false;
bool areSimilar(int[] one, int[] two)
{
//Cheat: yes, I went there
if (one.Length > 10000 || two.Length > 10000)
{ return true; }
//cheat
if (!ArraysContainSameValues(one, two))
{ return false; }
return TestSimilarity(one, two);
}
private bool TestSimilarity(int[] one, int[] two)
{
for (int position = 0; position < one.Length; position++)
{
if (one[position] == two[position])
{ continue; }
if (_OneMoved)
{
_OneMoved = false;
return false;
}
int AlternatePosition = Array.IndexOf(one, two[position]);
if (one[position] != two[AlternatePosition])
{ return false; }
_OneMoved = true;
return areSimilar(one, SwitchValues(two, position, AlternatePosition));
}
return true;
}
private int[] SwitchValues(int[] two, int position, int alternatePosition)
{
int temp = two[position];
two[position] = two[alternatePosition];
two[alternatePosition] = temp;
return two;
}
private bool ArraysContainSameValues(int[] one, int[] two)
{
foreach(int value in one)
{
if (!two.Contains(value))
{
return false;
}
int oneCount = ValueCount(one, value), twoCount = ValueCount(two, value);
if (ValueCount(one, value) != ValueCount(two, value))
{
return false;
}
}
return true;
}
private int ValueCount(int[] numberArray, int value)
{
int Count = 0;
foreach (int number in numberArray)
{
if (number == value)
{
Count++;
}
}
return Count;
}
arrayChange
You are given an array of integers. On each move you are allowed to increase exactly one of its element by one. Find the minimal number of moves required to obtain a strictly increasing sequence from the input.
Example
For inputArray = [1, 1, 1]
, the output should bearrayChange(inputArray) = 3
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Guaranteed constraints:
3 ≤ inputArray.length ≤ 105
,-105 ≤ inputArray[i] ≤ 105
. - [output] integer The minimal number of moves needed to obtain a strictly increasing sequence from
inputArray
.
It's guaranteed that for the given test cases the answer always fits signed32
-bit integer type.
int arrayChange(int[] inputArray)
{
int ShiftCount = 0;
for (int count = 0; count < inputArray.Length - 1; count++)
{
if (inputArray[count] < inputArray[count + 1])
{continue;}
int difference = inputArray[count] - inputArray[count + 1];
inputArray[count + 1] += difference + 1;
ShiftCount += difference + 1;
}
return ShiftCount;
}
palindromeRearranging
Given a string, find out if its characters can be rearranged to form a palindrome.
Example
For inputString = "aabb"
, the output should bepalindromeRearranging(inputString) = true
.
We can rearrange "aabb"
to make "abba"
, which is a palindrome.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A string consisting of lowercase English letters. Guaranteed constraints:
1 ≤ inputString.length ≤ 50
. - [output] boolean
true
if the characters of theinputString
can be rearranged to form a palindrome,false
otherwise.
bool palindromeRearranging(string inputString)
{
char OddCount = '\0';
foreach (char character in inputString)
{
if (inputString.Count(c => c == character) % 2 == 1)
{
if (IsEven(inputString))
{
return false;
}
if (OddCount != '\0' && OddCount != character) //Only one odd count allowed for odd numbered arrays
{
return false;
}
OddCount = character;
}
}
return true;
}
private bool IsEven(string inputString)
{
return inputString.Count() % 2 == 0;
}
Island of Knowledge
areEquallyStrong
Call two arms equally strong if the heaviest weights they each are able to lift are equal.
Call two people equally strong if their strongest arms are equally strong (the strongest arm can be both the right and the left), and so are their weakest arms.
Given your and your friend's arms' lifting capabilities find out if you two are equally strong.
Example
- For
yourLeft = 10
,yourRight = 15
,friendsLeft = 15
, andfriendsRight = 10
, the output should beareEquallyStrong(yourLeft, yourRight, friendsLeft, friendsRight) = true
; - For
yourLeft = 15
,yourRight = 10
,friendsLeft = 15
, andfriendsRight = 10
, the output should beareEquallyStrong(yourLeft, yourRight, friendsLeft, friendsRight) = true
; - For
yourLeft = 15
,yourRight = 10
,friendsLeft = 15
, andfriendsRight = 9
, the output should beareEquallyStrong(yourLeft, yourRight, friendsLeft, friendsRight) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer yourLeft A non-negative integer representing the heaviest weight you can lift with your left arm. Guaranteed constraints:
0 ≤ yourLeft ≤ 20
. - [input] integer yourRight A non-negative integer representing the heaviest weight you can lift with your right arm. Guaranteed constraints:
0 ≤ yourRight ≤ 20
. - [input] integer friendsLeft A non-negative integer representing the heaviest weight your friend can lift with his or her left arm. Guaranteed constraints:
0 ≤ friendsLeft ≤ 20
. - [input] integer friendsRight A non-negative integer representing the heaviest weight your friend can lift with his or her right arm. Guaranteed constraints:
0 ≤ friendsRight ≤ 20
. - [output] boolean
true
if you and your friend are equally strong,false
otherwise.
bool areEquallyStrong(int yourLeft, int yourRight, int friendsLeft, int friendsRight) {
if ( yourLeft == friendsLeft && yourRight == friendsRight)
{ return true; }
if (yourLeft == friendsRight && yourRight == friendsLeft)
{ return true; }
return false;
}
arrayMaximalAdjacentDifference
Given an array of integers, find the maximal absolute difference between any two of its adjacent elements.
Example
For inputArray = [2, 4, 1, 0]
, the output should bearrayMaximalAdjacentDifference(inputArray) = 3
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Guaranteed constraints:
3 ≤ inputArray.length ≤ 10
,-15 ≤ inputArray[i] ≤ 15
. - [output] integer The maximal absolute difference.
int arrayMaximalAdjacentDifference(int[] inputArray)
{
int RunningMax = 0;
for (int input = 0; input < inputArray.Length - 1; input++)
{
int CurrentDiff = Math.Abs(inputArray[input] - inputArray[input + 1]);
RunningMax = CurrentDiff > RunningMax ? CurrentDiff : RunningMax;
}
return RunningMax;
}
isIPv4Address
An IP address is a numerical label assigned to each device (e.g., computer, printer) participating in a computer network that uses the Internet Protocol for communication. There are two versions of the Internet protocol, and thus two versions of addresses. One of them is the IPv4 address.
Given a string, find out if it satisfies the IPv4 address naming rules.
Example
- For
inputString = "172.16.254.1"
, the output should beisIPv4Address(inputString) = true
; - For
inputString = "172.316.254.1"
, the output should beisIPv4Address(inputString) = false
.316
is not in range[0, 255]
. - For
inputString = ".254.255.0"
, the output should beisIPv4Address(inputString) = false
. There is no first number.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A string consisting of digits, full stops and lowercase English letters. Guaranteed constraints:
1 ≤ inputString.length ≤ 30
. - [output] boolean
true
ifinputString
satisfies the IPv4 address naming rules,false
otherwise.
bool isIPv4Address(string inputString)
{
List<char> Characters = inputString.ToList();
if (Characters.Count(c => c == '.') != 3)
{ return false; }
List<char> IPCantidate = new List<char>();
for (int character = 0, count = 0; character < Characters.Count; character++)
{
if (Characters[character] == '.')
{
if (IPCantidate.Count == 0)
{ return false; }
IPCantidate.Clear();
continue;
}
IPCantidate.Add(Characters[character]);
if (!int.TryParse(string.Concat(IPCantidate), out int number))
{ return false; }
if (number > 255 || number < 0)
{ return false; }
}
return true;
}
avoidObstacles
You are given an array of integers representing coordinates of obstacles situated on a straight line.
Assume that you are jumping from the point with coordinate 0
to the right. You are allowed only to make jumps of the same length represented by some integer.
Find the minimal length of the jump enough to avoid all the obstacles.
Example
For inputArray = [5, 3, 6, 7, 9]
, the output should beavoidObstacles(inputArray) = 4
.
Check out the image below for better understanding:

Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Non-empty array of positive integers. Guaranteed constraints:
2 ≤ inputArray.length ≤ 1000
,1 ≤ inputArray[i] ≤ 1000
. - [output] integer The desired length.
int avoidObstacles(int[] inputArray)
{
bool BadJump = false;
for (int jump = 2; jump <= inputArray.Max() + 1; jump++)
{
for (int position = 0; position <= inputArray.Max() + 1; position += jump)
{
if (inputArray.Contains(position))
{
BadJump = true;
break;
}
}
if (BadJump)
{
BadJump = false;
continue;
}
return jump;
}
return 0;
}
Box Blur
Last night you partied a little too hard. Now there's a black and white photo of you that's about to go viral! You can't let this ruin your reputation, so you want to apply the box blur algorithm to the photo to hide its content.
The pixels in the input image are represented as integers. The algorithm distorts the input image in the following way: Every pixel x
in the output image has a value equal to the average value of the pixel values from the 3 Ă— 3
square that has its center at x
, including x
itself. All the pixels on the border of x
are then removed.
Return the blurred image as an integer, with the fractions rounded down.
Example
For
image = [[1, 1, 1],
[1, 7, 1],
[1, 1, 1]]
the output should be boxBlur(image) = [[1]]
.
To get the value of the middle pixel in the input 3 Ă— 3
square: (1 + 1 + 1 + 1 + 7 + 1 + 1 + 1 + 1) = 15 / 9 = 1.66666 = 1
. The border pixels are cropped from the final result.
For
image = [[7, 4, 0, 1],
[5, 6, 2, 2],
[6, 10, 7, 8],
[1, 4, 2, 0]]
the output should be
boxBlur(image) = [[5, 4],
[4, 4]]
There are four 3 Ă— 3
squares in the input image, so there should be four integers in the blurred output. To get the first value: (7 + 4 + 0 + 5 + 6 + 2 + 6 + 10 + 7) = 47 / 9 = 5.2222 = 5
. The other three integers are obtained the same way, then the surrounding integers are cropped from the final result.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.array.integer image An image, stored as a rectangular matrix of non-negative integers. Guaranteed constraints:
3 ≤ image.length ≤ 100
,3 ≤ image[0].length ≤ 100
,0 ≤ image[i][j] ≤ 255
. - [output] array.array.integer A blurred image represented as integers, obtained through the process in the description.
int[][] boxBlur(int[][] image)
{
List<int[]> ReturnSet = new List<int[]>();
int MaxColumns = int.MaxValue;
for (int row = 0; row < image.Length; row++)
{
MaxColumns = image[row].Length < MaxColumns ? image[row].Length : MaxColumns;
}
for (int row0 = 0, row1 = 1, row2 = 2; row2 < image.Length; row0++, row1++, row2++)
{
List<int> Averages = new List<int>();
for (int column0 = 0, column1 = 1, column2 = 2; column2 < MaxColumns; column0++, column1++, column2++)
{
int sum =
image[row0][column0] + image[row0][column1] + image[row0][column2] +
image[row1][column0] + image[row1][column1] + image[row1][column2] +
image[row2][column0] + image[row2][column1] + image[row2][column2];
Averages.Add(sum / 9);
}
ReturnSet.Add(Averages.ToArray());
}
return ReturnSet.ToArray();
}
Minesweeper
In the popular Minesweeper game you have a board with some mines and those cells that don't contain a mine have a number in it that indicates the total number of mines in the neighboring cells. Starting off with some arrangement of mines we want to create a Minesweeper game setup.
Example
For
matrix = [[true, false, false],
[false, true, false],
[false, false, false]]
the output should be
minesweeper(matrix) = [[1, 2, 1],
[2, 1, 1],
[1, 1, 1]]
Check out the image below for better understanding:
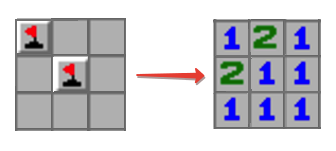
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.array.boolean matrix A non-empty rectangular matrix consisting of boolean values -
true
if the corresponding cell contains a mine,false
otherwise. Guaranteed constraints:2 ≤ matrix.length ≤ 100
,2 ≤ matrix[0].length ≤ 100
. - [output] array.array.integer Rectangular matrix of the same size as
matrix
each cell of which contains an integer equal to the number of mines in the neighboring cells. Two cells are called neighboring if they share at least one corner.
int[][] minesweeper(bool[][] matrix)
{
int[][] Sweeper = PrepMatrix(matrix);
for (int row = 0; row < matrix.Length; row++)
{
for (int column = 0; column < matrix[row].Length; column++)
{
bool RowMinus = row - 1 >= 0;
bool RowPlus = row + 1 < matrix.Length;
bool ColumnMinus = column - 1 >= 0;
bool ColumnPlus = column + 1 < matrix[row].Length;
if (RowMinus)
{
if (ColumnMinus)
{
if (matrix[row - 1][column - 1])
{ Sweeper[row][column]++; }
}
if (matrix[row - 1][column])
{ Sweeper[row][column]++; }
if (ColumnPlus)
{
if (matrix[row - 1][column + 1])
{ Sweeper[row][column]++; }
}
}
if (ColumnMinus)
{
if (matrix[row][column - 1])
{ Sweeper[row][column]++; }
}
if (ColumnPlus)
{
if (matrix[row][column + 1])
{ Sweeper[row][column]++; }
}
if (RowPlus)
{
if (ColumnMinus)
{
if (matrix[row + 1][column - 1])
{ Sweeper[row][column]++; }
}
if (matrix[row + 1][column])
{ Sweeper[row][column]++; }
if (ColumnPlus)
{
if (matrix[row + 1][column + 1])
{ Sweeper[row][column]++; }
}
}
}
}
return Sweeper;
}
int[][] PrepMatrix(bool[][] matrix)
{
List<int[]> rows = new List<int[]>();
for (int row = 0; row < matrix.Length; row++)
{
List<int> columns = new List<int>();
foreach (bool value in matrix[row])
{
columns.Add(0);
}
rows.Add(columns.ToArray());
}
return rows.ToArray();
}
Rains of Reason
Array Replace
Given an array of integers, replace all the occurrences of elemToReplace
with substitutionElem
.
Example
For inputArray = [1, 2, 1]
, elemToReplace = 1
, and substitutionElem = 3
, the output should bearrayReplace(inputArray, elemToReplace, substitutionElem) = [3, 2, 3]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Guaranteed constraints:
0 ≤ inputArray.length ≤ 104
,0 ≤ inputArray[i] ≤ 109
. - [input] integer elemToReplace Guaranteed constraints:
0 ≤ elemToReplace ≤ 109
. - [input] integer substitutionElem Guaranteed constraints:
0 ≤ substitutionElem ≤ 109
. - [output] array.integer
int[] arrayReplace(int[] inputArray, int elemToReplace, int substitutionElem)
{
for (int position = 0; position < inputArray.Length; position++)
{
if (inputArray[position] == elemToReplace)
{inputArray[position] = substitutionElem;}
}
return inputArray;
}
evenDigitsOnly
Check if all digits of the given integer are even.
Example
- For
n = 248622
, the output should beevenDigitsOnly(n) = true
; - For
n = 642386
, the output should beevenDigitsOnly(n) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n Guaranteed constraints:
1 ≤ n ≤ 109
. - [output] boolean
true
if all digits ofn
are even,false
otherwise.
bool evenDigitsOnly(int n)
{
while (n > 0)
{
if (n % 2 == 0)
{
n = (n - (n % 10)) / 10;
continue;
}
return false;
}
return true;
}
variableName
Correct variable names consist only of English letters, digits and underscores and they can't start with a digit.
Check if the given string is a correct variable name.
Example
- For
name = "var_1__Int"
, the output should bevariableName(name) = true
; - For
name = "qq-q"
, the output should bevariableName(name) = false
; - For
name = "2w2"
, the output should bevariableName(name) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string name Guaranteed constraints:
1 ≤ name.length ≤ 10
. - [output] boolean
true
ifname
is a correct variable name,false
otherwise.
bool variableName(string name)
{
if (name.All(c => (c >= 48 && c <= 57) || (c >= 65 && c <= 90) || c == 95 || (c >= 97 && c <= 122)))
{
if (name[0] > 57)
{
return true;
}
}
return false;
}
alphabeticShift
Given a string, your task is to replace each of its characters by the next one in the English alphabet; i.e. replace a
with b
, replace b
with c
, etc (z
would be replaced by a
).
Example
For inputString = "crazy"
, the output should be alphabeticShift(inputString) = "dsbaz"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A non-empty string consisting of lowercase English characters. Guaranteed constraints:
1 ≤ inputString.length ≤ 1000
. - [output] string The resulting string after replacing each of its characters.
string alphabeticShift(string inputString)
{
List<char> Characters = inputString.ToList();
for (int character = 0; character < Characters.Count; character++)
{
if (Characters[character] != 'z' && Characters[character] != 'Z')
{
Characters[character]++;
continue;
}
if (Characters[character] == 'z')
{ Characters[character] = 'a'; }
if (Characters[character] == 'Z')
{ Characters[character] = 'A'; }
}
return string.Concat(Characters);
}
chessBoardCellColor
Given two cells on the standard chess board, determine whether they have the same color or not.
Example
- For
cell1 = "A1"
andcell2 = "C3"
, the output should bechessBoardCellColor(cell1, cell2) = true
.
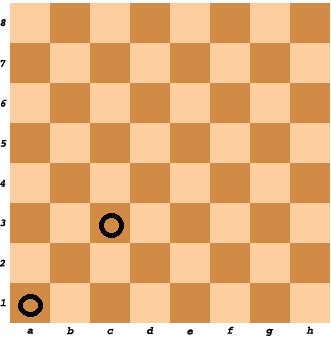
- For
cell1 = "A1"
andcell2 = "H3"
, the output should be chessBoardCellColor(cell1, cell2) = false
.
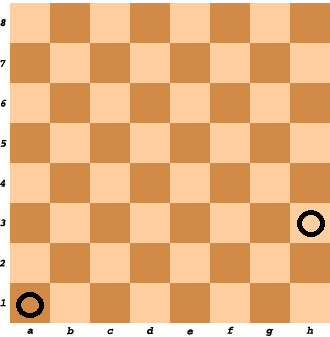
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string cell1 Guaranteed constraints:
cell1.length = 2
,'A' ≤ cell1[0] ≤ 'H'
,1 ≤ cell1[1] ≤ 8
. - [input] string cell2 Guaranteed constraints:
cell2.length = 2
,'A' ≤ cell2[0] ≤ 'H'
,1 ≤ cell2[1] ≤ 8
. - [output] boolean
true
if both cells have the same color,false
otherwise.
enum BoardColors { Black, White };
enum BoardRow { A, B, C, D, E, F, G, H, I};
bool chessBoardCellColor(string cell1, string cell2)
{
Dictionary<string, BoardColors> CheckeredBoard = BuildBoard();
return CheckeredBoard[cell1] == CheckeredBoard[cell2];
}
Dictionary<string, BoardColors> BuildBoard()
{
BoardColors PlaceHolder = BoardColors.Black;
Dictionary<string, BoardColors> CheckeredBoard = new Dictionary<string, BoardColors>();
for (BoardRow column = BoardRow.A; column < BoardRow.I; column++)
{
for (int row = 1; row <= 8; row++)
{
PlaceHolder = row == 1 ?
PlaceHolder :
PlaceHolder == BoardColors.Black ?
BoardColors.White : BoardColors.Black;
CheckeredBoard.Add(string.Concat(column.ToString(), row), PlaceHolder);
}
}
return CheckeredBoard;
}
Through The Fog
Circle of Numbers
Consider integer numbers from 0
to n - 1
written down along the circle in such a way that the distance between any two neighboring numbers is equal (note that 0
and n - 1
are neighboring, too).
Given n
and firstNumber
, find the number which is written in the radially opposite position to firstNumber
.
Example
For n = 10
and firstNumber = 2
, the output should besolution(n, firstNumber) = 7
.
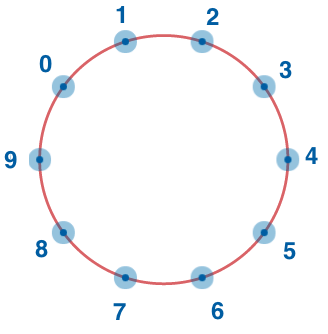
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n A positive even integer. Guaranteed constraints:
4 ≤ n ≤ 20
. - [input] integer firstNumber Guaranteed constraints:
0 ≤ firstNumber ≤ n - 1
. - [output] integer
int solution(int n, int firstNumber)
{
return firstNumber < (n / 2) ? firstNumber + (n/2) : firstNumber - (n / 2);
}
depositProfit
You have deposited a specific amount of money into your bank account. Each year your balance increases at the same growth rate
. With the assumption that you don't make any additional deposits, find out how long it would take for your balance to pass a specific threshold
.
Example
For deposit = 100
, rate = 20
, and threshold = 170
, the output should besolution(deposit, rate, threshold) = 3
.
Each year the amount of money in your account increases by 20%
. So throughout the years, your balance would be:
- year 0:
100
; - year 1:
120
; - year 2:
144
; - year 3:
172.8
.
Thus, it will take 3
years for your balance to pass the threshold
, so the answer is 3
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer deposit The initial deposit, guaranteed to be a positive integer. Guaranteed constraints:
1 ≤ deposit ≤ 100
. - [input] integer rate The rate of increase. Each year the balance increases by the
rate
percent of the current sum. Guaranteed constraints:1 ≤ rate ≤ 100
. - [input] integer threshold The target balance. Guaranteed constraints:
deposit < threshold ≤ 200
. - [output] integer The number of years it would take to hit the
threshold
.
int solution(int deposit, int rate, int threshold)
{
int year = 0;
double apr = (double)rate / 100;
double balance = deposit;
while (balance < threshold)
{
balance += balance * apr;
year++;
}
return year;
}
absoluteValuesSumMinimization
Given a sorted array of integers a
, your task is to determine which element of a
is closest to all other values of a
. In other words, find the element x
in a
, which minimizes the following sum:
abs(a[0] - x) + abs(a[1] - x) + ... + abs(a[a.length - 1] - x)
(where abs
denotes the absolute value)
If there are several possible answers, output the smallest one.
Example
- For
a = [2, 4, 7]
, the output should besolution(a) = 4
.- for
x = 2
, the value will beabs(2 - 2) + abs(4 - 2) + abs(7 - 2) = 7
. - for
x = 4
, the value will beabs(2 - 4) + abs(4 - 4) + abs(7 - 4) = 5
. - for
x = 7
, the value will beabs(2 - 7) + abs(4 - 7) + abs(7 - 7) = 8
.
x = 4
, so the answer is4
. - for
- For
a = [2, 3]
, the output should besolution(a) = 2
.- for
x = 2
, the value will beabs(2 - 2) + abs(3 - 2) = 1
. - for
x = 3
, the value will beabs(2 - 3) + abs(3 - 3) = 1
.
x
betweenx = 2
andx = 3
is the answer. - for
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer a A non-empty array of integers, sorted in ascending order. Guaranteed constraints:
1 ≤ a.length ≤ 1000
,-106 ≤ a[i] ≤ 106
. - [output] integer An integer representing the element from
a
that minimizes the sum of its absolute differences with all other elements.
int solution(int[] a)
{
int returnNumer = 0;
int LowestResult = int.MaxValue;
foreach(int testValue in a)
{
int sum = 0;
for (int count = 0; count < a.Length; count++)
{
sum += Math.Abs(a[count] - testValue);
}
if (sum < LowestResult)
{
returnNumer = testValue;
LowestResult = sum;
}
}
return returnNumer;
}
stringsRearrangement
Given an array of equal-length strings, you'd like to know if it's possible to rearrange the order of the elements in such a way that each consecutive pair of strings differ by exactly one character. Return true
if it's possible, and false
if not.
Note: You're only rearranging the order of the strings, not the order of the letters within the strings!
Example
- For
inputArray = ["aba", "bbb", "bab"]
, the output should besolution(inputArray) = false
. There are 6 possible arrangements for these strings:["aba", "bbb", "bab"]
["aba", "bab", "bbb"]
["bbb", "aba", "bab"]
["bbb", "bab", "aba"]
["bab", "bbb", "aba"]
["bab", "aba", "bbb"]
false
. - For
inputArray = ["ab", "bb", "aa"]
, the output should besolution(inputArray) = true
. It's possible to arrange these strings in a way that each consecutive pair of strings differ by 1 character (eg:"aa", "ab", "bb"
or"bb", "ab", "aa"
), so returntrue
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.string inputArray A non-empty array of strings of lowercase letters. Guaranteed constraints:
2 ≤ inputArray.length ≤ 10
,1 ≤ inputArray[i].length ≤ 15
. - [output] boolean Return
true
if the strings can be reordered in such a way that each neighbouring pair of strings differ by exactly one character (false
otherwise).
bool solution(string[] inputArray)
{
List<string> Input = inputArray.ToList();
return InProperOrder(Input) ? true : BruteForceCheck(Input);
}
private bool BruteForceCheck(List<string> input)
{
return GetPermutations(input);
}
private bool GetPermutations(List<string> currentList, int permuteIndex = 0)
{
if (InProperOrder(currentList))
{ return true; }
if (permuteIndex == currentList.Count)
{ return false; }
for (int count = 0; count < currentList.Count - 1; count++)
{
if (permuteIndex == count)
{ continue; }
if (GetPermutations(Swap(currentList, count, permuteIndex), permuteIndex + 1))
{ return true; }
}
return false;
}
private List<string> Swap(List<string> input, int value, int swap)
{
List<string> tList = input;
string temp = tList[value];
tList[value] = tList[swap];
tList[swap] = temp;
return tList;
}
private bool InProperOrder(List<string> input)
{
for (int value = 0; value < input.Count -1; value++)
{
int differences = 0;
for (int character = 0; character < input[value].Length; character++)
{
if (input[value][character] != input[value + 1][character])
{
differences++;
}
}
if (differences == 0 || differences > 1)
{ return false; }
}
return true;
}
private bool OneCharacterDifference(string candidate, string test)
{
int differences = 0;
for (int character = 0; character < candidate.Length; character++)
{
if (candidate[character] != test[character])
{ differences++; }
}
return differences == 1;
}
Diving Deeper
extractEachKth
Given array of integers, remove each kth
element from it.
Example
For inputArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
and k = 3
, the output should besolution(inputArray, k) = [1, 2, 4, 5, 7, 8, 10]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Guaranteed constraints:
5 ≤ inputArray.length ≤ 15
,-20 ≤ inputArray[i] ≤ 20
. - [input] integer k Guaranteed constraints:
1 ≤ k ≤ 10
. - [output] array.integer
inputArray
without elementsk - 1
,2k - 1
,3k - 1
etc.
int[] solution(int[] inputArray, int k)
{
List<int> input = inputArray.ToList();
for (int position = input.Count; position > 0; position--)
{
if (position % k != 0)
{ continue; }
input.RemoveAt(position - 1);
}
return input.ToArray();
}
firstDigit
Find the leftmost digit that occurs in a given string.
Example
- For
inputString = "var_1__Int"
, the output should besolution(inputString) = '1'
; - For
inputString = "q2q-q"
, the output should besolution(inputString) = '2'
; - For
inputString = "0ss"
, the output should besolution(inputString) = '0'
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A string containing at least one digit. Guaranteed constraints:
3 ≤ inputString.length ≤ 10
. - [output] char
char solution(string inputString)
{
return inputString.First(c => c >= '0' && c <= '9');
}
differentSymbolsNaive
Given a string, find the number of different characters in it.
Example
For s = "cabca"
, the output should besolution(s) = 3
.
There are 3
different characters a
, b
and c
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string s A string of lowercase English letters. Guaranteed constraints:
3 ≤ s.length ≤ 1000
. - [output] integer
int solution(string s)
{
Dictionary<char, int> Symbols = new Dictionary<char, int>();
foreach (char Character in s)
{
if (!Symbols.ContainsKey(Character))
{
Symbols.Add(Character, 1);
continue;
}
Symbols[Character]++;
}
return Symbols.Count();
}
arrayMaxConsecutiveSum
Given array of integers, find the maximal possible sum of some of its k
consecutive elements.
Example
For inputArray = [2, 3, 5, 1, 6]
and k = 2
, the output should besolution(inputArray, k) = 8
.
All possible sums of 2
consecutive elements are:
2 + 3 = 5
;3 + 5 = 8
;5 + 1 = 6
;1 + 6 = 7
.
Thus, the answer is8
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer inputArray Array of positive integers. Guaranteed constraints:
3 ≤ inputArray.length ≤ 105
,1 ≤ inputArray[i] ≤ 1000
. - [input] integer k An integer (not greater than the length of
inputArray
). Guaranteed constraints:1 ≤ k ≤ inputArray.length
. - [output] integer The maximal possible sum.
int solution(int[] inputArray, int k)
{
int maxSum = 0;
for (int value = 0; value < k ; value++)
{
maxSum += inputArray[value];
}
int rollingSum = maxSum;
for (int value = 0; value < inputArray.Length - k; value++)
{
rollingSum -= inputArray[value];
rollingSum += inputArray[value + k];
maxSum = maxSum < rollingSum ? rollingSum : maxSum;
}
return maxSum;
}
Dark Wilderness
Growing Plant
Caring for a plant can be hard work, but since you tend to it regularly, you have a plant that grows consistently. Each day, its height increases by a fixed amount represented by the integer upSpeed
. But due to lack of sunlight, the plant decreases in height every night, by an amount represented by downSpeed
.
Since you grew the plant from a seed, it started at height 0
initially. Given an integer desiredHeight
, your task is to find how many days it'll take for the plant to reach this height.
Example
For upSpeed = 100
, downSpeed = 10
, and desiredHeight = 910
, the output should besolution(upSpeed, downSpeed, desiredHeight) = 10
.
# | Day | Night |
---|---|---|
1 | 100 | 90 |
2 | 190 | 180 |
3 | 280 | 270 |
4 | 370 | 360 |
5 | 460 | 450 |
6 | 550 | 540 |
7 | 640 | 630 |
8 | 730 | 720 |
9 | 820 | 810 |
10 | 910 | 900 |
The plant first reaches a height of 910
on day 10
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer upSpeed A positive integer representing the daily growth of the plant. Guaranteed constraints:
3 ≤ upSpeed ≤ 100
. - [input] integer downSpeed A positive integer representing the nightly decline of the plant. Guaranteed constraints:
2 ≤ downSpeed < upSpeed
. - [input] integer desiredHeight A positive integer representing the goal height. Guaranteed constraints:
4 ≤ desiredHeight ≤ 1000
. - [output] integer The number of days that it will take for the plant to reach / pass
desiredHeight
.
int solution(int upSpeed, int downSpeed, int desiredHeight)
{
int Height = upSpeed;
int days = 1;
while (Height < desiredHeight)
{
Height -= downSpeed;
Height += upSpeed;
days++;
}
return days;
}
Knapsack Light
You found two items in a treasure chest! The first item weighs weight1
and is worth value1
, and the second item weighs weight2
and is worth value2
. What is the total maximum value of the items you can take with you, assuming that your max weight capacity is maxW
and you can't come back for the items later?
Note that there are only two items and you can't bring more than one item of each type, i.e. you can't take two first items or two second items.
Example
- For
value1 = 10
,weight1 = 5
,value2 = 6
,weight2 = 4
, andmaxW = 8
, the output should besolution(value1, weight1, value2, weight2, maxW) = 10
. You can only carry the first item. - For
value1 = 10
,weight1 = 5
,value2 = 6
,weight2 = 4
, andmaxW = 9
, the output should besolution(value1, weight1, value2, weight2, maxW) = 16
. You're strong enough to take both of the items with you. - For
value1 = 5
,weight1 = 3
,value2 = 7
,weight2 = 4
, andmaxW = 6
, the output should besolution(value1, weight1, value2, weight2, maxW) = 7
. You can't take both items, but you can take any of them.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer value1 Guaranteed constraints:
2 ≤ value1 ≤ 20
. - [input] integer weight1 Guaranteed constraints:
2 ≤ weight1 ≤ 10
. - [input] integer value2 Guaranteed constraints:
2 ≤ value2 ≤ 20
. - [input] integer weight2 Guaranteed constraints:
2 ≤ weight2 ≤ 10
. - [input] integer maxW Guaranteed constraints:
1 ≤ maxW ≤ 20
. - [output] integer
int solution(int value1, int weight1, int value2, int weight2, int maxW)
{
if (weight1 > maxW && weight2 > maxW)
{ return 0; }
if (weight1 + weight2 <= maxW)
{ return value1 + value2; }
if (weight1 <= maxW && weight2 <= maxW)
{ return value1 > value2 ? value1 : value2; }
if (weight1 > maxW)
{ return value2; }
return value1;
}
longestDigitsPrefix
Given a string, output its longest prefix which contains only digits.
Example
For inputString = "123aa1"
, the output should besolution(inputString) = "123"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString Guaranteed constraints:
3 ≤ inputString.length ≤ 100
. - [output] string
string solution(string inputString)
{
List<char> prefix = new List<char>();
foreach (char Character in inputString)
{
if (Character < '0' || Character > '9')
{ break; }
prefix.Add(Character);
}
return string.Concat(prefix);
}
digitDegree
digitDegree
Let's define digit degree of some positive integer as the number of times we need to replace this number with the sum of its digits until we get to a one digit number.
Given an integer, find its digit degree.
Example
- For
n = 5
, the output should besolution(n) = 0
; - For
n = 100
, the output should besolution(n) = 1
.1 + 0 + 0 = 1
. - For
n = 91
, the output should besolution(n) = 2
.9 + 1 = 10
->1 + 0 = 1
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n Guaranteed constraints:
5 ≤ n ≤ 109
. - [output] integer
int solution(int n)
{
int degrees = 0;
while (n > 9)
{
List<int> Digits = GetDigits(n);
n = Digits.Sum();
degrees++;
}
return degrees;
}
List<int> GetDigits(int number)
{
List<int> Digits = new List<int>();
while (number > 0)
{
Digits.Add(number % 10);
number = number / 10;
}
return Digits;
}
Bishop and Pawn
Bishop and Pawn
Given the positions of a white bishop
and a black pawn
on the standard chess board, determine whether the bishop can capture the pawn in one move.
The bishop has no restrictions in distance for each move, but is limited to diagonal movement. Check out the example below to see how it can move:
Example
- For
bishop = "a1"
andpawn = "c3"
, the output should besolution(bishop, pawn) = true
. - For
bishop = "h1"
andpawn = "h3"
, the output should besolution(bishop, pawn) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string bishop Coordinates of the white bishop in the chess notation. Guaranteed constraints:
bishop.length = 2
,'a' ≤ bishop[0] ≤ 'h'
,1 ≤ bishop[1] ≤ 8
. - [input] string pawn Coordinates of the black pawn in the same notation. Guaranteed constraints:
pawn.length = 2
,'a' ≤ pawn[0] ≤ 'h'
,1 ≤ pawn[1] ≤ 8
. - [output] boolean
true
if the bishop can capture the pawn,false
otherwise.
bool solution(string bishop, string pawn)
{
int VertPos = bishop[0] > pawn[0] ? pawn[0] - bishop[0] : bishop[0] - pawn[0];
int HorizPos = GetHorizontalPosition(bishop, pawn);
return Math.Abs(VertPos) == Math.Abs(HorizPos);
}
private int GetHorizontalPosition(string bishop, string pawn)
{
int HorizBish = int.Parse(bishop[1].ToString());
int HorizPawn = int.Parse(pawn[1].ToString());
return HorizBish > HorizPawn ? HorizPawn - HorizBish : HorizBish - HorizPawn;
}
Eruption of Light
isBeautifulString
isBeautifulString
A string is said to be beautiful if each letter in the string appears at most as many times as the previous letter in the alphabet within the string; ie: b
occurs no more times than a
; c
occurs no more times than b
; etc. Note that letter a
has no previous letter.
Given a string, check whether it is beautiful.
Example
- For
inputString = "bbbaacdafe"
, the output should besolution(inputString) = true
. This string contains 3a
s, 3b
s, 1c
, 1d
, 1e
, and 1f
(and 0 of every other letter), so since there aren't any letters that appear more frequently than the previous letter, this string qualifies as beautiful. - For
inputString = "aabbb"
, the output should besolution(inputString) = false
. Since there are moreb
s thana
s, this string is not beautiful. - For
inputString = "bbc"
, the output should besolution(inputString) = false
. Although there are moreb
s thanc
s, this string is not beautiful because there are noa
s, so therefore there are moreb
s thana
s.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString A string of lowercase English letters. Guaranteed constraints:
3 ≤ inputString.length ≤ 50
. - [output] boolean Return
true
if the string is beautiful,false
otherwise.
bool solution(string inputString)
{
Dictionary<char, int> Occurances = GetOccurences(inputString);
List<char> Keys = Occurances.Keys.ToList();
Keys.Sort();
if (!ContainsAllContiguous(Keys))
{ return false; }
for (int character = 1; character < Keys.Count; character++)
{
if (Occurances[Keys[character - 1]] < Occurances[Keys[character]])
{ return false; }
}
return true;
}
private bool ContainsAllContiguous(List<char> Keys)
{
for (int character = 'a'; character <= Keys[Keys.Count - 1]; character++)
{
if (!Keys.Contains((char)character))
{ return false; }
}
return true;
}
private Dictionary<char, int> GetOccurences(string inputString)
{
Dictionary<char, int> Occurrances = new Dictionary<char, int>();
foreach (char Character in inputString)
{
if (!Occurrances.ContainsKey(Character))
{
Occurrances.Add(Character, 1);
continue;
}
Occurrances[Character]++;
}
return Occurrances;
}
Find Email Domain
Find Email Domain
An email address such as "John.Smith@example.com"
is made up of a local part ("John.Smith"
), an "@"
symbol, then a domain part ("example.com"
).
The domain name part of an email address may only consist of letters, digits, hyphens and dots. The local part, however, also allows a lot of different special characters. Here you can look at several examples of correct and incorrect email addresses.
Given a valid email address, find its domain part.
Example
- For
address = "prettyandsimple@example.com"
, the output should besolution(address) = "example.com"
; - For
address = "fully-qualified-domain@codesignal.com"
, the output should besolution(address) = "codesignal.com"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string address Guaranteed constraints:
10 ≤ address.length ≤ 50
. - [output] string
string solution(string address)
{
return address.Remove(0, address.LastIndexOf('@')+ 1);
}
buildPalindrome
Given a string, find the shortest possible string which can be achieved by adding characters to the end of initial string to make it a palindrome.
Example
For st = "abcdc"
, the output should besolution(st) = "abcdcba"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string st A string consisting of lowercase English letters. Guaranteed constraints:
3 ≤ st.length ≤ 10
. - [output] string
string solution(string st)
{
if (Palindromic(st))
{
return st;
}
List<char> Balancers = new List<char>();
for (int left = 0; left < st.Count(); left++)
{
if (Palindromic(st, left)) //extends from the left only
{ break; }
Balancers.Add(st[left]);
}
Balancers.Reverse();
return string.Concat(st, string.Concat(Balancers));
}
private bool Palindromic(string characters, int start = 0)
{
for (int left = start, right = characters.Count() - 1; left < right; left++, right--)
{
if (characters[left] != characters[right])
{
return false;
}
}
return true;
}
Elections Winners
Elections are in progress!
Given an array of the numbers of votes given to each of the candidates so far, and an integer k
equal to the number of voters who haven't cast their vote yet, find the number of candidates who still have a chance to win the election.
The winner of the election must secure strictly more votes than any other candidate. If two or more candidates receive the same (maximum) number of votes, assume there is no winner at all.
Example
For votes = [2, 3, 5, 2]
and k = 3
, the output should besolution(votes, k) = 2
.
- The first candidate got
2
votes. Even if all of the remaining3
candidates vote for him, he will still have only5
votes, i.e. the same number as the third candidate, so there will be no winner. - The second candidate can win if all the remaining candidates vote for him (
3 + 3 = 6 > 5
). - The third candidate can win even if none of the remaining candidates vote for him. For example, if each of the remaining voters cast their votes for each of his opponents, he will still be the winner (the
votes
array will thus be[3, 4, 5, 3]
). - The last candidate can't win no matter what (for the same reason as the first candidate).
Thus, only 2
candidates can win (the second and the third), which is the answer.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.integer votes A non-empty array of non-negative integers. Its
ith
element denotes the number of votes cast for theith
candidate. Guaranteed constraints:4 ≤ votes.length ≤ 105
,0 ≤ votes[i] ≤ 104
. - [input] integer k The number of voters who haven't cast their vote yet. Guaranteed constraints:
0 ≤ k ≤ 105
. - [output] integer
int solution(int[] votes, int k)
{
int MaxVotes = votes.Max();
int CouldWin = votes.Count(v => v + k > MaxVotes);
return CouldWin > 0 ? CouldWin : votes.Count(v => v == MaxVotes) == 1 ? 1 : 0;
}
Is MAC48 Address?
A media access control address (MAC address) is a unique identifier assigned to network interfaces for communications on the physical network segment.
The standard (IEEE 802) format for printing MAC-48 addresses in human-friendly form is six groups of two hexadecimal digits (0
to 9
or A
to F
), separated by hyphens (e.g. 01-23-45-67-89-AB
).
Your task is to check by given string inputString
whether it corresponds to MAC-48 address or not.
Example
- For
inputString = "00-1B-63-84-45-E6"
, the output should besolution(inputString) = true
; - For
inputString = "Z1-1B-63-84-45-E6"
, the output should besolution(inputString) = false
; - For
inputString = "not a MAC-48 address"
, the output should besolution(inputString) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString Guaranteed constraints:
15 ≤ inputString.length ≤ 20
. - [output] boolean
true
ifinputString
corresponds to MAC-48 address naming rules,false
otherwise.
bool solution(string inputString)
{
List<string> elements = inputString.Split('-').ToList();
if (elements.Count != 6)
{ return false; }
foreach (string element in elements)
{
if (element.Length != 2)
{ return false; }
foreach(char character in element)
{
if(!int.TryParse(character.ToString(), out int ThrowAway))
{
if((character > 'f' && character <= 'z') || (character > 'F' && character <= 'Z'))
{ return false; }
}
}
}
return true;
}
Rainbow of Clarity
isDigit
Determine if the given character is a digit or not.
Example
- For
symbol = '0'
, the output should besolution(symbol) = true
; - For
symbol = '-'
, the output should besolution(symbol) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] char symbol A character which is either a digit or not. Guaranteed constraints:
Given symbol is from ASCII table.
- [output] boolean
true
ifsymbol
is a digit,false
otherwise.
bool solution(char symbol)
{
return Char.IsNumber(symbol);
}
lineEncoding
Given a string, return its encoding defined as follows:
- First, the string is divided into the least possible number of disjoint substrings consisting of identical characters
- for example,
"aabbbc"
is divided into["aa", "bbb", "c"]
- for example,
- Next, each substring with length greater than one is replaced with a concatenation of its length and the repeating character
- for example, substring
"bbb"
is replaced by"3b"
- for example, substring
- Finally, all the new strings are concatenated together in the same order and a new string is returned.
Example
For s = "aabbbc"
, the output should besolution(s) = "2a3bc"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string s String consisting of lowercase English letters. Guaranteed constraints:
4 ≤ s.length ≤ 15
. - [output] string Encoded version of
s
.
string solution(string s)
{
char current = '\0';
int count = 0;
List<string> output = new List<string>();
foreach (char character in s)
{
if (character != current)
{
if (current != '\0')
{
output.Add(count == 1 ? current.ToString() : string.Concat(count, current));
}
current = character;
count = 1;
continue;
}
count++;
}
output.Add(count == 1 ? current.ToString() : string.Concat(count, current)); //Catch the last occurrance counted
return string.Concat(output);
}
chessKnight
Given a position of a knight on the standard chessboard, find the number of different moves the knight can perform.
The knight can move to a square that is two squares horizontally and one square vertically, or two squares vertically and one square horizontally away from it. The complete move therefore looks like the letter L. Check out the image below to see all valid moves for a knight piece that is placed on one of the central squares.
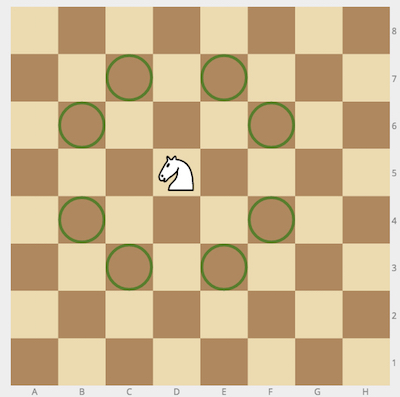
Example
- For
cell = "a1"
, the output should besolution(cell) = 2
. - For
cell = "c2"
, the output should besolution(cell) = 6
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string cell String consisting of 2 letters - coordinates of the knight on an
8 Ă— 8
chessboard in chess notation. Guaranteed constraints:cell.length = 2
,'a' ≤ cell[0] ≤ 'h'
,1 ≤ cell[1] ≤ 8
. - [output] integer
int solution(string cell)
{
cell.ToLower(); //just in case
char Column = cell[0];
int Row = int.Parse(cell[1].ToString());
if (Column >= 'c' && Column <= 'f')
{
if (Row >= 3 && Row <= 6)
{ return 8; }
if (Row == 2 || Row == 7)
{ return 6; }
if (Row == 1 || Row == 8)
{ return 4; }
}
if (Column == 'b' || Column == 'g')
{
if (Row >= 3 && Row <= 6)
{ return 6; }
if (Row == 2 || Row == 7)
{ return 4; }
if (Row == 1 || Row == 8)
{ return 2; }
}
if (Column == 'a' || Column == 'h')
{
if (Row >= 3 && Row <= 6)
{ return 4; }
if (Row == 2 || Row == 7)
{ return 3; }
if (Row == 1 || Row == 8)
{ return 2; }
}
return 0;
}
deleteDigit
Given some integer, find the maximal number you can obtain by deleting exactly one digit of the given number.
Example
- For
n = 152
, the output should besolution(n) = 52
; - For
n = 1001
, the output should besolution(n) = 101
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n Guaranteed constraints:
10 ≤ n ≤ 106
. - [output] integer
[C#] Syntax Tips
int solution(int n)
{
List<char> Number = n.ToString().ToList();
int MaxValue = 0;
for (int skip = 0; skip < Number.Count; skip++)
{
List<char> tNumber = new List<char>();
for (int digit = 0; digit < Number.Count; digit++)
{
if (digit != skip)
{ tNumber.Add(Number[digit]); }
}
int.TryParse(string.Concat(tNumber), out int tValue);
MaxValue = MaxValue > tValue ? MaxValue : tValue;
}
return MaxValue;
}
Land of Logic
longestWord
Define a word as a sequence of consecutive English letters. Find the longest word from the given string.
Example
For text = "Ready, steady, go!"
, the output should besolution(text) = "steady"
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string text Guaranteed constraints:
4 ≤ text.length ≤ 50
. - [output] string The longest word from
text
. It's guaranteed that there is a unique output.
string solution(string text)
{
List<string> Words = new List<string>();
List<char> characters = new List<char>();
for (int character = 0; character < text.Length; character++)
{
if (text[character] < 'A' || (text[character] > 'Z' && text[character] < 'a') || text[character] > 'z')
{
if (characters.Count > 0)
{
Words.Add(string.Concat(characters));
characters.Clear();
}
continue;
}
characters.Add(text[character]);
}
if (characters.Count > 0)
{ Words.Add(string.Concat(characters)); } //catches the last word if it was missed
string Longest = string.Empty;
foreach (string word in Words)
{
Longest = Longest.Length < word.Length ? word : Longest;
}
return Longest;
}
Valid Time
Check if the given string is a correct time representation of the 24-hour clock.
Example
- For
time = "13:58"
, the output should besolution(time) = true
; - For
time = "25:51"
, the output should besolution(time) = false
; - For
time = "02:76"
, the output should besolution(time) = false
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string time A string representing time in
HH:MM
format. It is guaranteed that the first two characters, as well as the last two characters, are digits. - [output] boolean
true
if the given representation is correct,false
otherwise.
bool solution(string time)
{
List<string> Parts = time.Split(':').ToList();
int.TryParse(Parts[0], out int Hours);
int.TryParse(Parts[1], out int minutes);
if (Hours >= 24)
{ return false; }
if (minutes >= 60)
{ return false; }
return true;
}
sumUpNumbers
CodeMaster has just returned from shopping. He scanned the check of the items he bought and gave the resulting string to Ratiorg to figure out the total number of purchased items. Since Ratiorg is a bot he is definitely going to automate it, so he needs a program that sums up all the numbers which appear in the given input.
Help Ratiorg by writing a function that returns the sum of numbers that appear in the given inputString
.
Example
For inputString = "2 apples, 12 oranges"
, the output should besolution(inputString) = 14
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string inputString Guaranteed constraints:
0 ≤ inputString.length ≤ 105
. - [output] integer
int solution(string inputString)
{
List<string> Numbers = new List<string>();
List<char> digits = new List<char>();
for (int character = 0; character < inputString.Length; character++)
{
if (!char.IsDigit(inputString[character]))
{
if (digits.Count() > 0)
{
Numbers.Add(string.Concat(digits));
digits.Clear();
continue;
}
}
digits.Add(inputString[character]);
}
if (digits.Count > 0)
{ Numbers.Add(string.Concat(digits)); } //catches any missed numbers
int total = 0;
foreach (string number in Numbers)
{
int.TryParse(number, out int temp); //i hate the word shouldn't
total += temp;
}
return total;
}
Different Squares
Given a rectangular matrix containing only digits, calculate the number of different 2 Ă— 2
squares in it.
Example
For
matrix = [[1, 2, 1],
[2, 2, 2],
[2, 2, 2],
[1, 2, 3],
[2, 2, 1]]
the output should besolution(matrix) = 6
.
Here are all 6
different 2 Ă— 2
squares:
- 1 2
2 2 - 2 1
2 2 - 2 2
2 2 - 2 2
1 2 - 2 2
2 3 - 2 3
2 1
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.array.integer matrix Guaranteed constraints:
1 ≤ matrix.length ≤ 100
,1 ≤ matrix[i].length ≤ 100
,0 ≤ matrix[i][j] ≤ 9
. - [output] integer The number of different
2 Ă— 2
squares inmatrix
.
int solution(int[][] matrix)
{
List<int[][]> Squares = new List<int[][]>();
for (int row = 0; row < matrix.Length - 1; row++)
{
for (int column = 0; column < matrix[row].Length - 1; column++)
{
int[][] square = new int[2][];
square[0] = new int[] { matrix[row][column], matrix[row][column + 1] };
square[1] = new int[] { matrix[row + 1][column], matrix[row + 1][column + 1] };
Squares.Add(square);
}
}
return CountNonDuplicates(Squares);
}
private int CountNonDuplicates(List<int[][]> squares)
{
List<int[][]> temp = new List<int[][]>();
for (int square = 0; square < squares.Count; square++)
{
bool duplicate = false;
for (int test = 0; test < temp.Count; test++)
{
if (squares[square][0][0] != temp[test][0][0])
{ continue; }
if (squares[square][0][1] != temp[test][0][1])
{ continue; }
if (squares[square][1][0] != temp[test][1][0])
{ continue; }
if (squares[square][1][1] != temp[test][1][1])
{ continue; }
duplicate = true;
}
if (!duplicate)
{
temp.Add(squares[square]);
}
}
return temp.Count();
}
digitsProduct
Given an integer product
, find the smallest positive (i.e. greater than 0
) integer the product of whose digits is equal to product
. If there is no such integer, return -1
instead.
Example
- For
product = 12
, the output should besolution(product) = 26
; - For
product = 19
, the output should besolution(product) = -1
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer product Guaranteed constraints:
0 ≤ product ≤ 600
. - [output] integer
int solution(int product)
{
if (product < 10 && product > 0)
{return product;}
//Hack: When product == 33 is wrong... 484 is an edge case that takes an inordinately long time to solve
if (IsPrime(product) || product == 33 || product == 484)
{ return -1; }
bool found = false;
int canditate = 1;
while (!found)
{
if (MultiplyDigits(canditate) == product)
{
found = true;
return canditate;
}
canditate++;
}
return -1;
}
private int MultiplyDigits(int canditate)
{
List<int> digits = new List<int>();
while (canditate > 0)
{
digits.Add(canditate % 10);
canditate /= 10;
}
int Product = 1;
foreach (int digit in digits)
{
Product *= digit;
}
return Product;
}
private bool IsPrime(int product)
{
if (product == 1)
{ return false; } //because 1 is included in the definition of prime, it (apparently) can not therefore be prime
List<int> tPrimes = new List<int>();
tPrimes.Add(2); //first prime to compare to;
for (int candidate = 3; candidate <= Math.Sqrt(product); candidate += 2)
{
bool prime = true;
foreach (int tPrime in tPrimes)
{
if (candidate % tPrime == 0)
{
prime = false;
break;
}
}
if (prime)
{
tPrimes.Add(candidate);
}
}
foreach (int tPrime in tPrimes)
{
if (product % tPrime == 0)
{ return false; }
}
return true;
}
File Naming
You are given an array of desired filenames in the order of their creation. Since two files cannot have equal names, the one which comes later will have an addition to its name in a form of (k)
, where k
is the smallest positive integer such that the obtained name is not used yet.
Return an array of names that will be given to the files.
Example
For names = ["doc", "doc", "image", "doc(1)", "doc"]
, the output should besolution(names) = ["doc", "doc(1)", "image", "doc(1)(1)", "doc(2)"]
.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.string names Guaranteed constraints:
5 ≤ names.length ≤ 1000
,1 ≤ names[i].length ≤ 15
. - [output] array.string
string[] solution(string[] names)
{
Dictionary<string, int> Files = new Dictionary<string, int>();
List<string> FileNames = new List<string>();
foreach (string name in names)
{
if (!FileNames.Contains(name))
{
Files.Add(name, 1);
FileNames.Add(name);
continue;
}
else
{
if (!Files.ContainsKey(name))
{
Files.Add(name, 1);
FileNames.Add(string.Format(string.Concat(name, "({0})"), Files[name]));
}
else
{
while (FileNames.Contains(String.Format(String.Concat(name, "({0})"), Files[name])))
{
Files[name]++;
}
FileNames.Add(string.Format(string.Concat(name, "({0})"), Files[name]));
}
}
}
return FileNames.ToArray();
}
messageFromBinaryCode
You are taking part in an Escape Room challenge designed specifically for programmers. In your efforts to find a clue, you've found a binary code written on the wall behind a vase, and realized that it must be an encrypted message. After some thought, your first guess is that each consecutive 8
bits of the code stand for the character with the corresponding extended ASCII code.
Assuming that your hunch is correct, decode the message.
Example
For code = "010010000110010101101100011011000110111100100001"
, the output should besolution(code) = "Hello!"
.
The first 8
characters of the code are 01001000
, which is 72
in the binary numeral system. 72
stands for H
in the ASCII-table, so the first letter is H
.
Other letters can be obtained in the same manner.
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] string code A string, the encrypted message consisting of characters
'0'
and'1'
. Guaranteed constraints:0 < code.length < 800
. - [output] string The decrypted message.
string solution(string code)
{
List<string> Bytes = Enumerable.Range(0, code.Length / 8).Select(i => code.Substring(i * 8, 8)).ToList();
List<char> Characters = GetCharacters(Bytes);
return string.Concat(Characters);
}
private List<char> GetCharacters(List<string> bytes)
{
List<char> Characters = new List<char>();
foreach(string Byte in bytes)
{
int Value = 0;
for (int digit = 0; digit < Byte.Length; digit++)
{
if (Byte[digit] == '1')
{
Value += ByteValue(digit + 1);
}
}
Characters.Add((char)Value);
}
return Characters;
}
private int ByteValue(int digit)
{
switch (digit)
{
case 8:
return 1;
case 7:
return 2;
case 6:
return 4;
case 5:
return 8;
case 4:
return 16;
case 3:
return 32;
case 2:
return 64;
case 1:
return 128;
default:
return 0;
}
}
spiralNumbers
Construct a square matrix with a size N Ă— N
containing integers from 1
to N * N
in a spiral order, starting from top-left and in clockwise direction.
Example
For n = 3
, the output should be
solution(n) = [[1, 2, 3],
[8, 9, 4],
[7, 6, 5]]
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] integer n Matrix size, a positive integer. Guaranteed constraints:
3 ≤ n ≤ 100
. - [output] array.array.integer
int[][] solution(int n)
{
int[][] Spiral = BuildEmpty(n);
int count = 1;
int row = 0;
bool rowIncrease = true;
int column = 0;
bool columnIncrease = true;
bool MoveHorizontal = true;
while (count <= n * n)
{
Spiral[row][column] = count;
count++;
if (MoveHorizontal)
{
if (columnIncrease)
{
if (column + 1 >= n)
{
columnIncrease = !columnIncrease;
MoveHorizontal = !MoveHorizontal;
row++;
continue;
}
if (Spiral[row][column + 1] != 0 )
{
columnIncrease = !columnIncrease;
MoveHorizontal = !MoveHorizontal;
row++;
continue;
}
column++;
continue;
}
else
{
if (column - 1 < 0)
{
columnIncrease = !columnIncrease;
MoveHorizontal = !MoveHorizontal;
row--;
continue;
}
if (Spiral[row][column - 1] != 0)
{
columnIncrease = !columnIncrease;
MoveHorizontal = !MoveHorizontal;
row--;
continue;
}
column--;
continue;
}
}
else
{
if (rowIncrease)
{
if (row + 1 >= n)
{
rowIncrease = !rowIncrease;
MoveHorizontal = !MoveHorizontal;
column--;
continue;
}
if (Spiral[row + 1][column] != 0)
{
rowIncrease = !rowIncrease;
MoveHorizontal = !MoveHorizontal;
column--;
continue;
}
row++;
continue;
}
else
{
if (row - 1 < 0)
{
rowIncrease = !rowIncrease;
MoveHorizontal = !MoveHorizontal;
column++;
continue;
}
if (Spiral[row - 1][column] != 0)
{
rowIncrease = !rowIncrease;
MoveHorizontal = !MoveHorizontal;
column++;
continue;
}
row--;
continue;
}
}
}
return Spiral;
}
private int[][] BuildEmpty(int n)
{
int[][] Empty =new int[n][];
for (int row = 0; row < n; row++)
{
Empty[row] = new int[n];
}
return Empty;
}
Sudoku
Sudoku is a number-placement puzzle. The objective is to fill a 9 Ă— 9
grid with digits so that each column, each row, and each of the nine 3 Ă— 3
sub-grids that compose the grid contains all of the digits from 1
to 9
.
This algorithm should check if the given grid of numbers represents a correct solution to Sudoku.
Example
- For
grid = [[1, 3, 2, 5, 4, 6, 9, 8, 7],
[4, 6, 5, 8, 7, 9, 3, 2, 1],
[7, 9, 8, 2, 1, 3, 6, 5, 4],
[9, 2, 1, 4, 3, 5, 8, 7, 6],
[3, 5, 4, 7, 6, 8, 2, 1, 9],
[6, 8, 7, 1, 9, 2, 5, 4, 3],
[5, 7, 6, 9, 8, 1, 4, 3, 2],
[2, 4, 3, 6, 5, 7, 1, 9, 8],
[8, 1, 9, 3, 2, 4, 7, 6, 5]]
the output should besolution(grid) = true
;
- For
grid = [[1, 3, 2, 5, 4, 6, 9, 2, 7],
[4, 6, 5, 8, 7, 9, 3, 8, 1],
[7, 9, 8, 2, 1, 3, 6, 5, 4],
[9, 2, 1, 4, 3, 5, 8, 7, 6],
[3, 5, 4, 7, 6, 8, 2, 1, 9],
[6, 8, 7, 1, 9, 2, 5, 4, 3],
[5, 7, 6, 9, 8, 1, 4, 3, 2],
[2, 4, 3, 6, 5, 7, 1, 9, 8],
[8, 1, 9, 3, 2, 4, 7, 6, 5]]
the output should besolution(grid) = false
.
The output should be false
: each of the nine 3 Ă— 3
sub-grids should contain all of the digits from 1
to 9
.
These examples are represented on the image below.
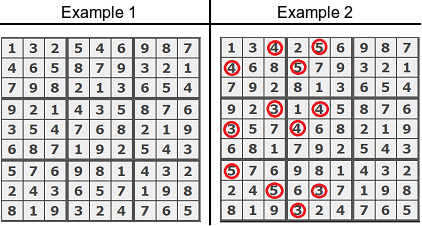
Input/Output
- [execution time limit] 3 seconds (cs)
- [input] array.array.integer grid A matrix representing
9 Ă— 9
grid already filled with numbers from1
to9
. Guaranteed constraints:grid.length = 9
,grid[i].length = 9
,1 ≤ grid[i][j] ≤ 9
. - [output] boolean
true
if the given grid represents a correct solution to Sudoku,false
otherwise.
bool solution(int[][] grid)
{
for (int row = 0; row < grid.Length; row++)
{
if (!Validate(grid[row].ToList()))
{ return false; }
}
List<List<int>> Columns = GetColumns(grid);
for (int column = 0; column < Columns.Count; column++)
{
if (!Validate(Columns[column]))
{ return false; }
}
List<List<int>> Squares = GetSquares(grid);
for (int square = 0; square < Squares.Count; square++)
{
if (!Validate(Squares[square]))
{ return false; }
}
return true;
}
private List<List<int>> GetSquares(int[][] grid)
{
List<List<int>> Squares = new List<List<int>>();
for (int rSquare = 0; rSquare < 9; rSquare += 3)
{
for (int cSquare = 0; cSquare < 9; cSquare += 3)
{
List<int> Square = new List<int>();
for (int row = 0; row < 3; row++)
{
for (int column = 0; column < 3; column++)
{
Square.Add(grid[rSquare + row][cSquare + column]);
}
}
Squares.Add(Square);
}
}
return Squares;
}
private List<List<int>> GetColumns(int[][] grid)
{
List<List<int>> columns = new List<List<int>>();
for (int column = 0; column < 9; column++)
{
List<int> tColumn = new List<int>();
for (int row = 0; row < grid.Length; row++)
{
tColumn.Add(grid[row][column]);
}
columns.Add(tColumn);
}
return columns;
}
private bool Validate(List<int> list)
{
if (!ValueSum(list))
{ return false; }
if (!OneEach(list))
{ return false; }
return true;
}
private bool OneEach(List<int> list)
{
List<int> Tally = new List<int>();
foreach (int value in list)
{
if (!Tally.Contains(value))
{
Tally.Add(value);
continue;
}
return false;
}
return true;
}
private bool ValueSum(List<int> list)
{
const int Actual = 45;
int Sum = 0;
foreach(int value in list)
{
Sum += value;
}
return Sum == Actual;
}
The Core
Intro Gates
Add Two Digits
You are given a two-digit integer n
. Return the sum of its digits.
Example
For n = 29
, the output should besolution(n) = 11
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n A positive two-digit integer. Guaranteed constraints:
10 ≤ n ≤ 99
. - [output] integer The sum of the first and second digits of the input number.
int solution(int n) {
return ((n % 10) + ((n - (n % 10)) / 10));
}
Largest Number
Given an integer n
, return the largest number that contains exactly n
digits.
Example
For n = 2
, the output should besolution(n) = 99
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n Guaranteed constraints:
1 ≤ n ≤ 9
. - [output] integer The largest integer of length
n
.
int solution(int n) {
return ((int)Math.Pow(10, n)) - 1;
}
Candies
n
children have got m
pieces of candy. They want to eat as much candy as they can, but each child must eat exactly the same amount of candy as any other child. Determine how many pieces of candy will be eaten by all the children together. Individual pieces of candy cannot be split.
Example
For n = 3
and m = 10
, the output should besolution(n, m) = 9
.
Each child will eat 3
pieces. So the answer is 9
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n The number of children. Guaranteed constraints:
1 ≤ n ≤ 10
. - [input] integer m The number of pieces of candy. Guaranteed constraints:
2 ≤ m ≤ 100
. - [output] integer The total number of pieces of candy the children will eat provided they eat as much as they can and all children eat the same amount.
int solution(int n, int m) {
return ((int)m/n) * n;
}
Seats in Theater
Your friend advised you to see a new performance in the most popular theater in the city. He knows a lot about art and his advice is usually good, but not this time: the performance turned out to be awfully dull. It's so bad you want to sneak out, which is quite simple, especially since the exit is located right behind your row to the left. All you need to do is climb over your seat and make your way to the exit.
The main problem is your shyness: you're afraid that you'll end up blocking the view (even if only for a couple of seconds) of all the people who sit behind you and in your column or the columns to your left. To gain some courage, you decide to calculate the number of such people and see if you can possibly make it to the exit without disturbing too many people.
Given the total number of rows and columns in the theater (nRows
and nCols
, respectively), and the row
and column
you're sitting in, return the number of people who sit strictly behind you and in your column or to the left, assuming all seats are occupied.
Example
For nCols = 16
, nRows = 11
, col = 5
, and row = 3
, the output should besolution(nCols, nRows, col, row) = 96
.
Here is what the theater looks like:
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer nCols An integer, the number of theater's columns. Guaranteed constraints:
1 ≤ nCols ≤ 1000
. - [input] integer nRows An integer, the number of theater's rows. Guaranteed constraints:
1 ≤ nRows ≤ 1000
. - [input] integer col An integer, the column number of your own seat (1-based). Guaranteed constraints:
1 ≤ col ≤ nCols
. - [input] integer row An integer, the row number of your own seat (1-based). Guaranteed constraints:
1 ≤ row ≤ nRows
. - [output] integer The number of people who sit strictly behind you and in your column or to the left.
int solution(int nCols, int nRows, int col, int row) {
return (nCols - col + 1) * (nRows - row);
}
Max Multiple
Given a divisor
and a bound
, find the largest integer N
such that:
N
is divisible bydivisor
.N
is less than or equal tobound
.N
is greater than0
.
It is guaranteed that such a number exists.
Example
For divisor = 3
and bound = 10
, the output should besolution(divisor, bound) = 9
.
The largest integer divisible by 3
and not larger than 10
is 9
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer divisor Guaranteed constraints:
2 ≤ divisor ≤ 10
. - [input] integer bound Guaranteed constraints:
5 ≤ bound ≤ 100
. - [output] integer The largest integer not greater than
bound
that is divisible bydivisor
.
int solution(int divisor, int bound) {
for (var count = bound; count > bound - divisor; count--)
{
if (count % divisor == 0)
{
return count;
}
}
return 0;
}
Circle of Numbers
Consider integer numbers from 0
to n - 1
written down along the circle in such a way that the distance between any two neighboring numbers is equal (note that 0
and n - 1
are neighboring, too).
Given n
and firstNumber
, find the number which is written in the radially opposite position to firstNumber
.
Example
For n = 10
and firstNumber = 2
, the output should besolution(n, firstNumber) = 7
.
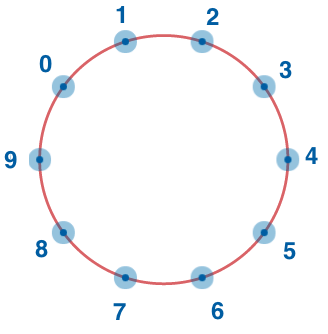
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n A positive even integer. Guaranteed constraints:
4 ≤ n ≤ 20
. - [input] integer firstNumber Guaranteed constraints:
0 ≤ firstNumber ≤ n - 1
. - [output] integer
int solution(int n, int firstNumber) {
if (firstNumber + (n/2) < n)
{
return firstNumber + (n/2);
}
return firstNumber - (n/2);
}
Late Ride
One night you go for a ride on your motorcycle. At 00:00
you start your engine, and the built-in timer automatically begins counting the length of your ride, in minutes. Off you go to explore the neighborhood.
When you finally decide to head back, you realize there's a chance the bridges on your route home are up, leaving you stranded! Unfortunately, you don't have your watch on you and don't know what time it is. All you know thanks to the bike's timer is that n
minutes have passed since 00:00
.
Using the bike's timer, calculate the current time. Return an answer as the sum of digits that the digital timer in the format hh:mm
would show.
Example
- For
n = 240
, the output should besolution(n) = 4
. Since240
minutes have passed, the current time is04:00
. The digits sum up to0 + 4 + 0 + 0 = 4
, which is the answer. - For
n = 808
, the output should besolution(n) = 14
.808
minutes mean that it's13:28
now, so the answer should be1 + 3 + 2 + 8 = 14
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n The duration of your ride, in minutes. It is guaranteed that you've been riding for less than a day (24 hours). Guaranteed constraints:
0 ≤ n < 60 · 24
. - [output] integer The sum of the digits the digital timer would show.
int solution(int n) {
int minutes = n % 60;
int hours = (n - minutes) / 60;
minutes = sumDigits(minutes);
hours = sumDigits(hours);
return minutes + hours;
}
int sumDigits(int digits)
{
int ones = digits % 10;
return (ones + ((digits - ones) / 10));
}
Phone Call
Some phone usage rate may be described as follows:
- first minute of a call costs
min1
cents, - each minute from the 2nd up to 10th (inclusive) costs
min2_10
cents - each minute after 10th costs
min11
cents.
You have s
cents on your account before the call. What is the duration of the longest call (in minutes rounded down to the nearest integer) you can have?
Example
For min1 = 3
, min2_10 = 1
, min11 = 2
, and s = 20
, the output should besolution(min1, min2_10, min11, s) = 14
.
Here's why:
- the first minute costs
3
cents, which leaves you with20 - 3 = 17
cents; - the total cost of minutes
2
through10
is1 * 9 = 9
, so you can talk9
more minutes and still have17 - 9 = 8
cents; - each next minute costs
2
cents, which means that you can talk8 / 2 = 4
more minutes.
Thus, the longest call you can make is 1 + 9 + 4 = 14
minutes long.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer min1 Guaranteed constraints:
1 ≤ min1 ≤ 10
. - [input] integer min2_10 Guaranteed constraints:
1 ≤ min2_10 ≤ 10
. - [input] integer min11 Guaranteed constraints:
1 ≤ min11 ≤ 10
. - [input] integer s Guaranteed constraints:
2 ≤ s ≤ 500
. - [output] integer
int solution(int min1, int min2_10, int min11, int s) {
int call = 0;
if (s >= min1)
{
int LeftOver = s - min1;
call++;
if (LeftOver > 9 * min2_10)
{
LeftOver = LeftOver - (9 * min2_10);
call += 9;
call += FindLeftOver(LeftOver, min11);
}
else
{
call += FindLeftOver(LeftOver, min2_10);
}
}
return call;
}
int FindLeftOver(int leftOver, int cost)
{
return (leftOver - (leftOver % cost)) / cost;
}
At the Crossroads
Reach Next Level
You are playing an RPG game. Currently your experience points (XP) total is equal to experience
. To reach the next level your XP should be at least at threshold
. If you kill the monster in front of you, you will gain more experience points in the amount of the reward
.
Given values experience
, threshold
and reward
, check if you reach the next level after killing the monster.
Example
- For
experience = 10
,threshold = 15
, andreward = 5
, the output should besolution(experience, threshold, reward) = true
; - For
experience = 10
,threshold = 15
, andreward = 4
, the output should besolution(experience, threshold, reward) = false
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer experience Guaranteed constraints:
3 ≤ experience ≤ 250
. - [input] integer threshold Guaranteed constraints:
5 ≤ threshold ≤ 300
. - [input] integer reward Guaranteed constraints:
2 ≤ reward ≤ 65
. - [output] boolean
true
if you reach the next level,false
otherwise.
bool solution(int experience, int threshold, int reward) {
return (threshold - experience) <= reward;
}
Knapsack Light
You found two items in a treasure chest! The first item weighs weight1
and is worth value1
, and the second item weighs weight2
and is worth value2
. What is the total maximum value of the items you can take with you, assuming that your max weight capacity is maxW
and you can't come back for the items later?
Note that there are only two items and you can't bring more than one item of each type, i.e. you can't take two first items or two second items.
Example
- For
value1 = 10
,weight1 = 5
,value2 = 6
,weight2 = 4
, andmaxW = 8
, the output should besolution(value1, weight1, value2, weight2, maxW) = 10
. You can only carry the first item. - For
value1 = 10
,weight1 = 5
,value2 = 6
,weight2 = 4
, andmaxW = 9
, the output should besolution(value1, weight1, value2, weight2, maxW) = 16
. You're strong enough to take both of the items with you. - For
value1 = 5
,weight1 = 3
,value2 = 7
,weight2 = 4
, andmaxW = 6
, the output should besolution(value1, weight1, value2, weight2, maxW) = 7
. You can't take both items, but you can take any of them.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer value1 Guaranteed constraints:
2 ≤ value1 ≤ 20
. - [input] integer weight1 Guaranteed constraints:
2 ≤ weight1 ≤ 10
. - [input] integer value2 Guaranteed constraints:
2 ≤ value2 ≤ 20
. - [input] integer weight2 Guaranteed constraints:
2 ≤ weight2 ≤ 10
. - [input] integer maxW Guaranteed constraints:
1 ≤ maxW ≤ 20
. - [output] integer
int solution(int value1, int weight1, int value2, int weight2, int maxW)
{
if (weight1 + weight2 <= maxW) { return value1 + value2; }
if (weight1 <= maxW && weight2 <= maxW && weight1 + weight2 > maxW){return value1 > value2 ? value1 : value2;}
if (weight2 > maxW && weight1 <= maxW) { return value1; }
if (weight1 > maxW && weight2 <= maxW) { return value2; }
return 0;
}
Extra Number
You're given three integers, a
, b
and c
. It is guaranteed that two of these integers are equal to each other. What is the value of the third integer?
Example
For a = 2
, b = 7
, and c = 2
, the output should besolution(a, b, c) = 7
.
The two equal numbers are a
and c
. The third number (b
) equals 7
, which is the answer.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer a Guaranteed constraints:
1 ≤ a ≤ 109
. - [input] integer b Guaranteed constraints:
1 ≤ b ≤ 109
. - [input] integer c Guaranteed constraints:
1 ≤ c ≤ 109
. - [output] integer
int solution(int a, int b, int c) {
return a == b ? c : a == c ? b : b == c ? a : 0;
}
Is Infinite Process?
Given integers a
and b
, determine whether the following pseudocode results in an infinite loop
while a is not equal to b do
increase a by 1
decrease b by 1
Assume that the program is executed on a virtual machine which can store arbitrary long numbers and execute forever.
Example
- For
a = 2
andb = 6
, the output should besolution(a, b) = false
; - For
a = 2
andb = 3
, the output should besolution(a, b) = true
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer a Guaranteed constraints:
0 ≤ a ≤ 20
. - [input] integer b Guaranteed constraints:
0 ≤ b ≤ 20
. - [output] boolean
true
if the pseudocode will never stop,false
otherwise.
bool solution(int a, int b) {
if (a > b) return true;
if (a < b) return (b - a) % 2 == 1;
if (a == b) return false;
return false;
}
Arithmetic Expression
Consider an arithmetic expression of the form a#b=c
. Check whether it is possible to replace #
with one of the four signs: +
, -
, *
or /
to obtain a correct expression.
Example
- For
a = 2
,b = 3
, andc = 5
, the output should besolution(a, b, c) = true
. We can replace#
with a+
to obtain2 + 3 = 5
, so the answer istrue
. - For
a = 8
,b = 2
, andc = 4
, the output should besolution(a, b, c) = true
. We can replace#
with a/
to obtain8 / 2 = 4
, so the answer istrue
. - For
a = 8
,b = 3
, andc = 2
, the output should besolution(a, b, c) = false
.8 + 3 = 11 ≠2
;8 - 3 = 5 ≠2
;8 * 3 = 24 ≠2
;8 / 3 = 2.(6) ≠2
.
false
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer a Guaranteed constraints:
1 ≤ a ≤ 20
. - [input] integer b Guaranteed constraints:
1 ≤ b ≤ 20
. - [input] integer c Guaranteed constraints:
0 ≤ c ≤ 20
. - [output] boolean
true
if the desired replacement is possible,false
otherwise.
bool solution(int a, int b, int c) {
return (a + b == c) || (a - b == c) || (a * b == c) || ((float)a / b == c);
}
Tennis Set
In tennis, the winner of a set is based on how many games each player wins. The first player to win 6
games is declared the winner unless their opponent had already won 5
games, in which case the set continues until one of the players has won 7
games.
Given two integers score1
and score2
, your task is to determine if it is possible for a tennis set to be finished with a final score of score1
: score2
.
Example
- For
score1 = 3
andscore2 = 6
, the output should besolution(score1, score2) = true
. Since player 1 hadn't reached5
wins, the set ends once player 2 has won6
games. - For
score1 = 8
andscore2 = 5
, the output should besolution(score1, score2) = false
. Since both players won at least5
games, the set would've ended once one of them won the7th
one. - For
score1 = 6
andscore2 = 5
, the output should besolution(score1, score2) = false
. This set will continue until one of these players wins their7th
game, so this can't be the final score.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer score1 Number of games won by the
1st
player, non-negative integer. Guaranteed constraints:0 ≤ score1 ≤ 10
. - [input] integer score2 Number of games won by the
2nd
player, non-negative integer. Guaranteed constraints:0 ≤ score2 ≤ 10
. - [output] boolean
true
ifscore1 : score2
represents a possible score for an ended set,false
otherwise.
bool solution(int score1, int score2)
{
return score1 >= score2 ? CompareScore(score1, score2) : CompareScore(score2, score1);
}
private bool CompareScore(int score1, int score2)
{
if (score1 == score2) return false;
if (score2 < 5 && score1 == 6) { return true; }
if (score2 >= 5 && score1 == 7) { return true; }
return false;
}
Will You?
Once Mary heard a famous song, and a line from it stuck in her head. That line was "Will you still love me when I'm no longer young and beautiful?". Mary believes that a person is loved if and only if he/she is both young and beautiful, but this is quite a depressing thought, so she wants to put her belief to the test.
Knowing whether a person is young
, beautiful
and loved
, find out if they contradict Mary's belief.
A person contradicts Mary's belief if one of the following statements is true:
- they are
young
andbeautiful
but notloved
; - they are
loved
but notyoung
or notbeautiful
.
Example
- For
young = true
,beautiful = true
, andloved = true
, the output should besolution(young, beautiful, loved) = false
. Young and beautiful people are loved according to Mary's belief. - For
young = true
,beautiful = false
, andloved = true
, the output should besolution(young, beautiful, loved) = true
. Mary doesn't believe that not beautiful people can be loved.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] boolean young
- [input] boolean beautiful
- [input] boolean loved
- [output] boolean
true
if the person contradicts Mary's belief,false
otherwise.
bool solution(bool young, bool beautiful, bool loved) {
if ((!young || !beautiful) && !loved) { return false; }
if ((young && beautiful) && loved) { return false; }
return true;
}
Metro Card
You just bought a public transit card that allows you to ride the Metro for a certain number of days.
Here is how it works: upon first receiving the card, the system allocates you a 31
-day pass, which equals the number of days in January. The second time you pay for the card, your pass is extended by 28
days, i.e. the number of days in February (note that leap years are not considered), and so on. The 13th
time you extend the pass, you get 31
days again.
You just ran out of days on the card, and unfortunately you've forgotten how many times your pass has been extended so far. However, you do remember the number of days you were able to ride the Metro during this most recent month. Figure out the number of days by which your pass will now be extended, and return all the options as an array sorted in increasing order.
Example
For lastNumberOfDays = 30
, the output should besolution(lastNumberOfDays) = [31]
.
There are 30
days in April, June, September and November, so the next months to consider are May, July, October or December. All of them have exactly 31
days, which means that you will definitely get a 31
-days pass the next time you extend your card.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer lastNumberOfDays A positive integer, the number of days for which the card was extended the last time. Guaranteed constraints:
lastNumberOfDays = 28 or lastNumberOfDays = 30 or lastNumberOfDays = 31
. - [output] array.integer An array of positive integers, the possible number of days for which you will extend your pass. The elements of the array can only be equal to
28
,30
or31
and must be sorted in increasing order.
int[] solution(int lastNumberOfDays) {
if (lastNumberOfDays == 31) { return new int[] { 28, 30, 31 }; }
if (lastNumberOfDays == 28 || lastNumberOfDays == 30) { return new int[] {31}; }
return new int[] {};
}
Corner of 0s and 1s
Kill K-th Bit
Implement the missing code, denoted by ellipses. You may not modify the pre-existing code.
In order to stop the Mad Coder evil genius you need to decipher the encrypted message he sent to his minions. The message contains several numbers that, when typed into a supercomputer, will launch a missile into the sky blocking out the sun, and making all the people on Earth grumpy and sad.
You figured out that some numbers have a modified single digit in their binary representation. More specifically, in the given number n
the kth
bit from the right was initially set to 0
, but its current value might be different. It's now up to you to write a function that will change the kth
bit of n
back to 0
.
Example
- For
n = 37
andk = 3
, the output should besolution(n, k) = 33
.3710 = 1001012 ~> 1000012 = 3310
. - For
n = 37
andk = 4
, the output should besolution(n, k) = 37
. The4th
bit is0
already (looks like the Mad Coder forgot to encrypt this number), so the answer is still37
.
Input/Output
- [execution time limit] 0.5 seconds (cs)
- [input] integer n Guaranteed constraints:
0 ≤ n ≤ 231 - 1
. - [input] integer k The
1
-based index of the changed bit (counting from the right). Guaranteed constraints:1 ≤ k ≤ 31
. - [output] integer
int solution(int n, int k)
{
return ...;
}
int solution(int n, int k)
{
return ~(~n | (1<<k-1));
}
Databases
Welcome to the Table
project List
Your boss wants to identify the successful projects running in your company, so he asked you to prepare a list of all the currently active projects and their average monthly income.
You have stored the information about these projects in a simple database with a single Projects table that has five columns:
- internal_id: the company's internal identifier for the project;
- project_name: the official name of the project;
- team_size: the number of employees working on the project;
- team_lead: the name of the project manager;
- income: the average monthly income of the project.
Your boss says that internal project ids are irrelevant to him and that he isn't interested in how big the teams are. Since that's the case, he wants you to create another table by removing the internal_id and team_size columns from the existing Projects table. Return it sorted by internal_id
in ascending order.
Example
For the following table Projects
internal_id | project_name | team_size | team_lead | income |
---|---|---|---|---|
1384 | MapReduce | 100 | Jeffrey Dean | 0 |
2855 | Windows | 1000 | Bill Gates | 100500 |
5961 | Snapchat | 3 | Evan Spiegel | 2000 |
the resulting table should be
project_name | team_lead | income |
---|---|---|
MapReduce | Jeffrey Dean | 0 |
Windows | Bill Gates | 100500 |
Snapchat | Evan Spiegel | 2000 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
SELECT project_name, team_lead, income
FROM Projects
ORDER BY internal_id;
END
countriesSelection
Your friend wants to become a professional tour guide and travel all around the world. In pursuit of this dream, she enrolled in tour guide school. The professors in this school turned out to be very demanding, and one of them gave your friend a difficult assignment that she has to finish over the weekend.
Here's the assignment: Given a list of countries, your friend should identify all the countries that are in Africa. To help her, you have decided to write a function that will find all such countries from any set of countries. The countries table in which the countries are stored has the following structure:
name
: the name of the country;continent
: the continent on which the country is situated;population
: the country's population.
Your task is to return a new table that has the same columns, but that only contains the countries from Africa. The countries should be sorted alphabetically by their names.
Example
For the following table countries
name | continent | population |
---|---|---|
Austria | Europe | 8767919 |
Belize | North America | 375909 |
Botswana | Africa | 2230905 |
Cambodia | Asia | 15626444 |
Cameroon | Africa | 22709892 |
the output should be
name | continent | population |
---|---|---|
Botswana | Africa | 2230905 |
Cameroon | Africa | 22709892 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select * from countries where continent = 'Africa';
END
monthlyScholarships
Students at your university get scholarships that are paid out throughout the year.
Information about the scholarships is stored in the table scholarships, which has the structure:
id
: the unique student id;scholarship
: the amount of the annual scholarship the student has been awarded.
Now you need to calculate the amount of money each student should get per month. Given the table scholarships, build the resulting table as follows: The table should have the same columns as the initial table, but the scholarship
column should contain the amount of the student's monthly scholarship payout. The rows should be ordered by the students' id
s.
Example
For the following table scholarships
id | scholarship |
---|---|
1 | 12000 |
2 | 18000 |
3 | 24000 |
4 | 15000 |
5 | 21000 |
6 | 13000 |
the output should be
id | scholarship |
---|---|
1 | 1000 |
2 | 1500 |
3 | 2000 |
4 | 1250 |
5 | 1750 |
6 | 1083.3333333333333 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
id
, (scholarship / 12) as scholarship
from scholarships;
END
projectTeam
You've been promoted and assigned to a new project. The problem is, you don't know who you are working with and your predecessor has vanished without a trace! Luckily, each project in your company keeps its own activity database, which you are going to use to find out the names of your new colleagues.
Information about the project's activity is stored in table projectLog, which has the following structure:
id
: unique action id;name
: the name of the person who performed the action;description
: the description of the action;timestamp
: the timestamp of the action.
You only have access to the project's most recent history, but this should be enough for you. You've decided that finding everyone who interacted with the project in this period is the best way to start.
Given the table projectLog, build a new results table with a single name
column that contains the names of the project's contributors sorted in ascending order.
Example
For the following table projectLog
id | name | description | timestamp |
---|---|---|---|
1 | James Smith | add new logo | 2015-11-10 15:24:32 |
2 | John Johnson | update license | 2015-11-10 15:50:01 |
3 | John Johnson | fix typos | 2015-11-10 15:55:01 |
4 | James Smith | update logo | 2015-11-10 17:53:04 |
5 | James Smith | delete old logo | 2015-11-10 17:54:04 |
6 | Michael Williams | fix the build | 2015-11-12 13:37:00 |
7 | Mary Troppins | add new feature | 2015-11-08 17:54:04 |
8 | James Smith | fix fonts | 2015-11-14 13:54:04 |
9 | Richard Young | remove unneeded files | 2015-11-14 00:00:00 |
10 | Michael Williams | add tests | 2015-11-09 11:53:00 |
the output should be
name |
---|
James Smith |
John Johnson |
Mary Troppins |
Michael Williams |
Richard Young |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select distinct name from projectLog order by name;
END
automaticNotifications
Implement the missing code, denoted by ellipses. You may not modify the pre-existing code.
The application you've been working on for the past year is a huge success! It already has a large and active user community. You know the ID number, username, and email of each user. Each user also has a specific role that shows their position in the community. Information about the users is stored in the database as a table users, which has the following structure:
id
: the unique user ID;username
: the username of the user;role
the user's role;email
: the user's email.
You want to send users automatic notifications to let them know about the most recent updates. However, not all users should get these notifications: Administrators don't need notifications since they know about the updates already, and premium users don't need them since they get personalized weekly updates.
Given the users table, your task is to return the emails of all the users who should get notifications, i.e. those whose role
is not equal to "admin"
or "premium"
. Note that roles are case insensitive, so users with role
s of "Admin"
, "pReMiUm"
, etc. should also be excluded.
The resulting table should contain a single email
column and be sorted by email
s in ascending order.
Example
For the following table users
id | username | role | |
---|---|---|---|
6 | fasalytch | premium | much.premium@role.com |
13 | luckygirl | regular | fun@meh.com |
16 | todayhumor | guru | today@humor.com |
23 | Felix | admin | felix@codesignal.com |
52 | admin666 | AdmiN | iamtheadmin@admin.admin |
87 | solver100500 | regular | email@notbot.com |
the resulting table should be
email@notbot.com |
fun@meh.com |
today@humor.com |
The only three users who should get notifications are luckygirl
, todayhumor
, and solver100500
. Their emails are fun@meh.com
, today@humor.com
, and email@notbot.com
respectively, which should be sorted as email@notbot.com
, fun@meh.com
, and today@humor.com
.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
SELECT email
FROM users
WHERE ... ("admin", "premium")
ORDER BY email;
CREATE PROCEDURE solution()
SELECT email
FROM users
WHERE role not in ("admin", "premium")
ORDER BY email;
Always Leave Table in ORDER
volleyballResults
You are creating a website that will help you and your friends keep track of the results of volleyball teams from all around the world. Your website regularly crawls the web searching for new games, and adds the results of these games to the results table stored in your local database. After each update, the table should be sorted in ascending order by the total number of games won. This year's results are quite marvelous - at any given moment there are no two teams that have won the same number of games!
The results table contains the following columns:
name
- the unique name of the team;country
- the country of the team;scored
- the number of scored goals;missed
- the number of missed goals;wins
- the total number of games the team has won.
Your task is to sort the given results table in ascending order by the number of wins
.
Example
For given table results
name | country | scored | missed | wins |
---|---|---|---|---|
FC Tokyo | Japan | 26 | 28 | 1 |
Fujian | China | 24 | 26 | 0 |
Jesus Maria | Argentina | 25 | 23 | 3 |
University Blues | Australia | 16 | 25 | 2 |
the output should be
name | country | scored | missed | wins |
---|---|---|---|---|
Fujian | China | 24 | 26 | 0 |
FC Tokyo | Japan | 26 | 28 | 1 |
University Blues | Australia | 16 | 25 | 2 |
Jesus Maria | Argentina | 25 | 23 | 3 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select * from results order by wins;
END
mostExpensive
Mr. Cash wants to keep track of his expenses, so he has prepared a list of all the products he bought this month. Now he is interested in finding the product on which he spent the largest amount of money. If there are products that cost the same amount of money, he'd like to find the one with the lexicographically smallest name.
The list of expenses is stored in a table Products which has the following columns:
id
: unique product id;name
: the unique name of the product;price
: the price for one item;quantity
: the number of items bought.
The resulting table should contain one row with a single column: the product with the lexicographically smallest name on which Mr. Cash spent the largest amount of money.
The total amount of money spent on a product is calculated as price * quantity
.
Example
- For the following table Products
id | name | price | quantity |
---|---|---|---|
1 | MacBook Air | 1500 | 1 |
2 | Magic Mouse | 79 | 1 |
3 | Spray cleaner | 10 | 3 |
the output should be
name |
---|
MacBook Air |
- For the following table Products
id | name | price | quantity |
---|---|---|---|
1 | Tomato | 10 | 4 |
2 | Cucumber | 8 | 5 |
3 | Red Pepper | 20 | 2 |
4 | Feta | 40 | 1 |
the output should be
name |
---|
Cucumber |
While the total cost for each product was 40
, Cucumber
has the lexicographically smallest name.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select name from (select id, name, price * quantity as cost from Products) as p order by cost desc, name limit 1;
END
contestLeaderboard
You are working as a recruiter at a big IT company, and you're actively looking for candidates who take the top places in major programming contests. Since the grand finale of the annual City Competition, you've been reaching out to the top participants from the leaderboard, and successfully so.
You have already interviewed all the prize winners (the top 3
participants), but that's not enough right now. Your company needs more specialists, so now you would like to connect with the participants who took the next 5
places.
The contest leaderboard is stored in a table leaderboard with the following columns:
id
: unique id of the participant;name
: the name of the participant;score
: the score the participant achieved in the competition.
The resulting table should contain the names of the participants who took the 4th
to 8th
places inclusive, sorted in descending order of their places. If there are fewer than 8
participants, the results should contain those who ranked lower than 3rd
place.
It is guaranteed that there are at least 3 prize winners in the leaderboard and that all participants have different scores.
Example
For the following table leaderboard
id | name | score |
---|---|---|
1 | gongy | 3001 |
2 | urandom | 2401 |
3 | eduardische | 2477 |
4 | Gassa | 2999 |
5 | bcc32 | 2658 |
6 | Alex_2oo8 | 6000 |
7 | mirosuaf | 2479 |
8 | Sparik | 2399 |
9 | thomas_holmes | 2478 |
10 | cthaeghya | 2400 |
the output should be
name |
---|
bcc32 |
mirosuaf |
thomas_holmes |
eduardische |
urandom |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
/*create temporary table T (select * from leaderboard order by score desc limit 3);*/
select lb.name
from leaderboard lb
/*left join T on (T.id = lb.ID) and (select count(*) from leaderboard) > 8
where T.id is null*/
order by lb.score desc
limit 3, 5;
/*drop table T;*/
END
gradeDistribution
At the end of every semester your professor for "Introduction to Databases" saves the exam results of every student in a simple database system. In the database table Grades, there are five columns:
- Name: the name of the student;
- ID: the student's ID number (a 5 byte positive integer);
- Midterm1: the result of the first midterm out of 100 points;
- Midterm2: the result of the second midterm out of 100 points;
- Final: the result of the final exam, this time out of a possible 200 points.
According to school policy, there are three possible ways to evaluate a grade:
- Option 1:
- Midterm 1: 25% of the grade
- Midterm 2: 25% of the grade
- Final exam: 50% of the grade
- Option 2:
- Midterm 1: 50% of the grade
- Midterm 2: 50% of the grade
- Option 3:
- Final exam: 100% of the grade.
Each student's final grade comes from the option that works the best for that student.
As a Teaching Assistant (TA), you need to query the name and id of all the students whose best grade comes from Option 3, sorted based on the first 3 characters of their name. If the first 3 characters of two names are the same, then the student with the lower ID value comes first.
Example
For the following table Grades
Name | ID | Midterm1 | Midterm2 | Final |
David | 42334 | 34 | 54 | 124 |
Anthony | 54528 | 100 | 10 | 50 |
Jonathan | 58754 | 49 | 58 | 121 |
Jonty | 11000 | 25 | 30 | 180 |
Output should be
Name | ID |
David | 42334 |
Jonty | 11000 |
Jonathan | 58754 |
For David, Jonty and Jonathan, the best option is number 3. But Anthony's best option is the second one, because Option1 = 25% of 100 + 25% of 10 +50% of 50 = 52.5, Option2 = 50% of 100 + 50% of 10 = 55, Option3 = 100% of 50 = 50.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
create temporary table Options
(select name
,id
,(midterm1 * .25) + (midterm2 * .25) + (final * .5) as option1
,(midterm1 * .5) + (midterm2 * .5) as option2
,final
from Grades);
select Name, ID
from Options
where final > option1
and final > option2
order by left (name, 3), id;
drop table Options;
END
mischievousNephews
Your nephews Huey, Dewey, and Louie are staying with you over the winter holidays. Ever since they arrived, you've hardly had a day go by without some kind of incident - the little rascals do whatever they please! Actually, you're not even mad; the ideas they come up with are pretty amazing, and it looks like there's even a system to their mischief.
You decided to track and analyze their behavior, so you created the mischief table in your local database. The table has the following columns:
mischief_date
: the date of the mischief (of thedate
type);author
: the nephew who caused the mischief ("Huey"
,"Dewey"
or"Louie"
);title
: the title of the mischief.
It looks like each of your nephews is active on a specific day of the week. You decide to check your theory by creating another table as follows:
The resulting table should contain four columns, weekday
, mischief_date
, author
, and title
, where weekday
is the weekday of mischief_date
(0
for Monday, 1
for Tuesday, and so on, with 6
for Sunday). The table should be sorted by the weekday
column, and for each weekday
Huey's mischief should go first, Dewey's should go next, and Louie's should go last. In case of a tie, mischief_date
should be a tie-breaker. If there's still a tie, the record with the lexicographically smallest title
should go first.
It is guaranteed that all entries of mischief are unique.
Example
For the following table mischief
mischief_date | author | title |
---|---|---|
2016-12-01 | Dewey | Cook the golden fish in a bucket |
2016-12-01 | Dewey | Paint the walls pink |
2016-12-01 | Huey | Eat all the candies |
2016-12-01 | Louie | Wrap the cat in toilet paper |
2016-12-08 | Louie | Play hockey on linoleum |
2017-01-01 | Huey | Smash a window |
2017-02-06 | Dewey | Create a rink on the porch |
the output should be
weekday | mischief_date | author | title |
---|---|---|---|
0 | 2017-02-06 | Dewey | Create a rink on the porch |
3 | 2016-12-01 | Huey | Eat all the candies |
3 | 2016-12-01 | Dewey | Cook the golden fish in a bucket |
3 | 2016-12-01 | Dewey | Paint the walls pink |
3 | 2016-12-01 | Louie | Wrap the cat in toilet paper |
3 | 2016-12-08 | Louie | Play hockey on linoleum |
6 | 2017-01-01 | Huey | Smash a window |
The first and the eighth of December are Thursdays, the sixth of February is a Monday, and the first of January is a Sunday.
The dates in the example are given in the format YYYY-MM-DD
.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
create temporary table tMischief (
select case when dayofweek(mischief_date) != 1 then dayofweek(mischief_date) - 2
else 6
end as weekday
, case when author = 'Huey' then 0
when author = 'Dewey' then 1
when author = 'Louie' then 2
end as author_order
,mischief_date
,author
,title
from mischief
);
select weekday, mischief_date, author, title
from tMischief
order by weekday, author_order, mischief_date, title;
drop table tMischief;
END
Would you LIKE the Second Meal?
suspectsInvestigation
A large amount of money was stolen today from the main city bank, and as the chief of police it's your duty to find the robber.
You store information about your suspects in the table Suspect, which has the structure:
id
: unique suspect id;name
: suspect first name;surname
: suspect surname;height
: suspect height;weight
: suspect weight.
You have already gathered some evidence and discovered the following clues:
- according to the camera records, the robber is not taller than
170cm
; - the robber left their signature near the crime scene:
"B. Gre?n"
."B"
definitely stands for the first letter of robber's name, and"Gre?n"
is their surname. The4th
letter of the surname is smudged by ketchup and is unreadable.
To make the list of suspects smaller, you would like to filter out the suspects who can't possibly be guilty according to the information obtained from the clues. For each remaining suspect, you want to save his/her id
, name
and surname
. Please note that the information obtained from the clue should be considered case-insensitive, so for example "bill Green"
, and "Bill green"
, and "Bill Green"
should all be included in the new table.
Given the table Suspect, build the resulting table as follows: the table should have columns id
, name
and surname
and its values should be ordered by the suspects' id
s in ascending order.
Example
For the following table Suspect
id | name | surname | height | weight |
---|---|---|---|---|
1 | John | Doe | 165 | 60 |
2 | Bill | Green | 170 | 67 |
3 | Baelfire | Grehn | 172 | 70 |
4 | Bill | Gretan | 165 | 55 |
5 | Brendon | Grewn | 150 | 50 |
6 | bill | Green | 169 | 50 |
the output should be
id | name | surname |
---|---|---|
2 | Bill | Green |
5 | Brendon | Grewn |
6 | bill | Green |
The name of the 1st
suspect doesn't start with "B"
, the 3rd
suspect is taller than 170cm
, and the surname of the 4th
suspect doesn't match the given pattern, meaning that these suspects are not included in the results.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select id, name, surname
from Suspect
where height <= 170
and name like 'B%'
and surname like 'Gre_n';
END
suspectsInvestigation2
A large amount of money was stolen today from the main city bank, and as the chief of police it's your duty to find the robber.
You store information about your suspects in the table Suspect, which has the structure:
id
: unique suspect id;name
: suspect first name;surname
: suspect surname;height
: suspect height;weight
: suspect weight.
You have already gathered some evidence and discovered the following clues:
- according to the camera records, the robber is taller than
170cm
; - the robber left their signature near the crime scene:
"B. Gre?n"
."B"
definitely stands for the first letter of robber's name, and"Gre?n"
is their surname. The4th
letter of the surname is smudged by ketchup and is unreadable.
The clues you've obtained allow you to let some suspects go since they can't possibly be guilty, so now you need to build a list that contains the people who can be freed based on the gathered information. For each of these people, you need to know his/her id
, name
and surname
. Please note that the information obtained from the clue should be considered case-insensitive, so for example "bill Green"
, "Bill GrEeN"
, and "Bill Green"
should all be included in the new table.
Given the table Suspect, build the resulting table as follows: the table should have columns id
, name
and surname
and its values should be ordered by the suspects' id
s in ascending order.
Example
For the following table of Suspect
id | name | surname | height | weight |
---|---|---|---|---|
1 | John | Doe | 165 | 60 |
2 | Bill | Green | 170 | 67 |
3 | Baelfire | Grehn | 172 | 70 |
4 | Bill | Gretan | 185 | 55 |
5 | Brendon | Grewn | 180 | 50 |
6 | bill | Green | 172 | 50 |
the output should be
id | name | surname |
1 | John | Doe |
2 | Bill | Green |
4 | Bill | Gretan |
The 1st
and the 2nd
suspects are not taller than 170cm
, and the 4th
suspect's surname doesn't match the "Gre?n"
pattern, so these suspects can't be guilty.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select id, name, surname
from Suspect
where height <= 170
or name not like 'B%'
or surname not like 'Gre_n'
order by id;
END
securityBreach
You are managing a large website that uses a special algorithm for user identification. In particular, it generates a unique attribute for each person based only on their first and last names and some additional metadata.
After analyzing the server logs today you found out that the website security has been breached and the data of some of your users might have been compromised.
The users' info is stored in the table users with the following structure:
first_name
: user's first name;second_name
: user's last name;attribute
: a unique attribute string of this user.
It seems that only the users those attribute
was generated by the old version of your special algorithm were affected. Such attributes have the following format (accurate to letter cases): <one or more arbitrary character>%<first name>_<second name>%<zero or more arbitrary characters>
. It's your duty now to warn the users that have these attributes about possible risks.
Given the users table, compose the resulting table consisting only of the rows that contain affected users' info. The result should be sorted by the attribute
s in ascending order.
Example
For the following table users
first_name | second_name | attribute |
---|---|---|
Mikel | Cover | %Mikel_Cover% |
Vicenta | Kravitz | 0%Vicenta_Kravitz% |
Tosha | Cover | 02VO1aJ767GF7L186lpIfBR0fQ5406Q02YcpG42LDF4Bv26 |
Shayne | Dahlquist | 0R0V331K8Q7ypBi4Az3B6Nm0jCqUk%Shayne_Dahlquist%46E3O0u7t7 |
Carrol | Llanes | 2mDIb1SdJne5wfH1Al32BE92r7j1d60PJ263b2vyPn3zxQ2P7sVOM26J11UT6W0Np |
Lizabeth | Cover | d1gM87S0NEHp386jXOk0aDc7w8bx4u8q7D82ff2Z4YT43iLyZ39xYbEDXMk |
Mack | Chace | fAnU49nMrmGu308627J7ne3qqqSPJDnq6dwW607lahNB5DinTR2Rkp549G7 |
Vicenta | Marchese | kUJ3N67vLB07mQL9Ai7p18cXGzjdT32r8283ZQi |
Mikel | Kravitz | PBX86iw1Ied87Z9OarE6sdSLdt%Mikel_Kravitz%W73XOY9YaOgi060r2x12D2EmD7 |
Deirdre | Chace | PBX86iw1Ied87Z9OarE6sdSLdtDeirdrelChaceW73XOY9YaOgi060r2x12D2EmD7 |
Josphine | Arzate | PwWD95BCKVYn5YD7iHLMa3HjP9tH%josphine_arzate%d2hNHNd3RpqfUREN47 |
Deirdre | Chace | ryCE5FIyS8q54A5036luzVS91j6C7P76E9X0O58htzgthuX24LG%DEirdre_Chace% |
Julietta | Beer | Tn35g5h51u7ltW946J |
the output should be
first_name | second_name | attribute |
---|---|---|
Vicenta | Kravitz | 0%Vicenta_Kravitz% |
Shayne | Dahlquist | 0R0V331K8Q7ypBi4Az3B6Nm0jCqUk%Shayne_Dahlquist%46E3O0u7t7 |
Mikel | Kravitz | PBX86iw1Ied87Z9OarE6sdSLdt%Mikel_Kravitz%W73XOY9YaOgi060r2x12D2EmD7 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select *
from users
where attribute like binary concat('%_\%', first_name, '\_', second_name, '\%%')
order by attribute;
END
testCheck
Implement the missing code, denoted by ellipses. You may not modify the pre-existing code.
Your professor gave the class a bonus task: Write a program that will check the answers for the latest test. The program will be given a table answers with the following columns:
id
- the unique ID of the question;correct_answer
- the correct answer to the question, given as a string;given_answer
- the answer given to the question, which can be NULL.
Your task is to return the table with a column id
and a column checks
, where for each answers id
the following string should be returned:
"no answer"
if thegiven_answer
is empty;"correct"
if thegiven_answer
is the same as thecorrect_answer
;"incorrect"
if thegiven_answer
is not empty and is incorrect.
Order the records in the answer table by id
.
Example
For given table answers
id | correct_answer | given_answer |
---|---|---|
1 | a | a |
2 | b | NULL |
3 | c | b |
the output should be
id | checks |
---|---|
1 | correct |
2 | no answer |
3 | incorrect |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
SELECT id, IF (...) AS checks
FROM answers
ORDER BY id;
CREATE PROCEDURE solution()
SELECT id, IF ( given_answer = correct_answer, 'correct', (if (given_answer is null, 'no answer', 'incorrect')) ) AS checks
FROM answers
ORDER BY id;
expressionsVerification
You're a math teacher at an elementary school. Today you taught your class basic arithmetic operations ("+"
, "-"
, "*"
, "/"
) and now you need to give the students some homework. You have a lot of expressions in the format a <operation> b = c
, where a
, b
, and c
are some integers and operation
is one of the operations given above.
Information about these expressions is stored in the table expressions, which has the structure:
id
: the unique operation id;a
: an integer;b
: an integer;operation
: one of the operations given above ("+"
,"-"
,"*"
, or"/"
);c
: an integer.
The homework you're going to give is simple: For each expression, the student needs to determine whether it's correct or not, i.e. whether it's true that the expression to the left of the =
sign equals c
.
Since you have many students and checking all their answers manually is a lot of work, you want to streamline the process by automatically identifying all the expressions that are correct. Given the table expressions, build the resulting table as follows: The table should have the same columns as the initial table does, but it should only contain those rows that represent correct expressions. The rows should be ordered by id
.
Example
For the following table expressions
id | a | b | operation | c |
---|---|---|---|---|
1 | 2 | 3 | + | 5 |
2 | 2 | 3 | + | 6 |
3 | 3 | 2 | / | 1 |
4 | 4 | 7 | * | 28 |
5 | 54 | 2 | - | 27 |
6 | 3 | 0 | / | 0 |
the output should be
id | a | b | operation | c |
---|---|---|---|---|
1 | 2 | 3 | + | 5 |
4 | 4 | 7 | * | 28 |
Explanation:
- 2 + 3 = 5 - correct;
- 2 + 3 = 6 - incorrect;
- 3 / 2 = 1 - incorrect;
- 4 * 7 = 28 - correct;
- 54 - 2 = 27 - incorrect;
- 3 / 0 = 0 - incorrect.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select *
from expressions
where (operation = '+'
and c = a + b)
or (operation = '-'
and c = a - b)
or (operation = '*'
and c = a * b)
or (operation = '/'
and c = a / b);
END
newsSubscribers
You are managing a small newspaper subscription service. Anyone who uses it can subscribe to a large number of different newspapers for a full year or just a half year.
The information about subscriptions is stored in the full_year and half_year tables, which have the following structures:
- full_year:
id
: the unique subscription ID;newspaper
: the newspaper's name;subscriber
: the name of the subscriber.
- half_year
id
: the unique subscription ID;newspaper
: the newspaper's name;subscriber
: the name of the subscriber.
Given the full_year and half_year tables, compose the result as follows: The resulting table should have one column subscriber
that contains all the distinct names of anyone who is subscribed to a newspaper with the word Daily
in its name. The table should be sorted in ascending order by the subscribers' first names.
Example
The following tables full_year
id | newspaper | subscriber |
---|---|---|
1 | The Paragon Herald | Crissy Sepe |
2 | The Daily Reporter | Tonie Moreton |
3 | Morningtide Daily | Erwin Chitty |
4 | Daily Breakfast | Tonie Moreton |
5 | Independent Weekly | Lavelle Phu |
and half_year
id | newspaper | subscriber |
---|---|---|
1 | The Daily Reporter | Lavelle Phu |
2 | Daily Breakfast | Tonie Moreton |
3 | The Paragon Herald | Lia Cover |
4 | The Community Gazette | Lavelle Phu |
5 | Nova Daily | Lia Cover |
6 | Nova Daily | Joya Buss |
the output should be
subscriber |
---|
Erwin Chitty |
Joya Buss |
Lavelle Phu |
Lia Cover |
Tonie Moreton |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select subscriber
from full_year
where newspaper like '%daily%'
union
select subscriber
from half_year
where newspaper like '%daily%'
order by subscriber;
END
GROUP Dishes BY Type
countriesInfo
Your friend wants to become a professional tour guide and travel all around the world. In pursuit of this dream, she enrolled in tour guide school. The professors in this school turned out to be very demanding, and one of them gave your friend a difficult assignment that she has to finish over the weekend.
Here's the task: Given a list of countries, your friend should calculate the average population and total population of all the countries in the list. To help her, you have decided to write a function that will calculate the required values for any number of countries. The countries table in which the countries are stored has the following structure:
name
: the name of the country;continent
: the continent on which the country is situated;population
: the population of the country.
Your task is to return a new table that contains the number of countries in the given list, along with their average and total population, in columns titled number
, average
and total
.
Example
For the following table countries
name | continent | population |
---|---|---|
Grenada | North America | 103328 |
Monaco | Europe | 38400 |
San Marino | Europe | 33005 |
Vanuatu | Australia | 277500 |
the output should be
number | average | total |
---|---|---|
4 | 113058.2500 | 452233 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
count(*) as number
,sum(population) / count(*) as average
,sum(population) as total
from countries;
END
itemsCounts
You recently started working in the IT department of a large store. You were put in charge of the inventory database availableItems, which has the following structure:
id
: unique item ID;item_name
: the name of the item;item_type
: the type of the item.
Note that it is possible for items that are of different types to have the same names.
One of the most common operations performed on this database is querying the number of specific items available at the store. Since the database is quite large, queries of this type can take up too much time. You have decided to start analyzing this problem by counting the number of each available item.
Given the availableItems table, write a select statement which returns the following three columns: item_name
, item_type
and item_count
, containing the names of the items, their types, and the amount of those items, respectively. The output should be sorted in ascending order by item type, with items of the same type sorted in ascending order by their names.
Example
For the following table availableItems
id | item_name | item_type |
---|---|---|
1 | SafeDisk 4GB | USB drive |
2 | SafeDisk 8GB | USB drive |
3 | Cinco 50-Pack | DVD |
4 | SafeDisk 4GB | Memory card |
5 | SafeDisk 8GB | Memory card |
6 | Cinco 30-Pack | DVD |
7 | SafeDisk 4GB | Memory card |
8 | SafeDisk 4GB | Memory card |
9 | DiskHolder | Misc |
10 | Cinco 50-Pack | DVD |
11 | SafeDisk 4GB | USB drive |
12 | DiskCleaner Pro | Misc |
the output should be
item_name | item_type | item_count |
---|---|---|
Cinco 30-Pack | DVD | 1 |
Cinco 50-Pack | DVD | 2 |
SafeDisk 4GB | Memory card | 3 |
SafeDisk 8GB | Memory card | 1 |
DiskCleaner Pro | Misc | 1 |
DiskHolder | Misc | 1 |
SafeDisk 4GB | USB drive | 2 |
SafeDisk 8GB | USB drive | 1 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
item_name
,item_type
,count(*) as item_count
from availableItems
group by item_name, item_type
order by item_type, item_name;
END
usersByContinent
You are curious about the geographical distribution of CodeSignal users, so you have created a list of countries along with the number of registered users from each. Your task now is to calculate the number of users on each continent.
The information about the countries is stored in a table countries, which has 3
columns:
country
: the name of the country;continent
: the name of the continent where the country is located;users
: the number of users registered on CodeSignal in the country.
The answer should be a table with 2
columns, continent
and users
, sorted by the number of users in decreasing order.
Example
For the following table countries
country | continent | users |
---|---|---|
Armenia | Europe | 1000 |
France | Europe | 1300 |
Russia | Europe | 3000 |
USA | North America | 5000 |
the resulting table should be
continent | users |
---|---|
Europe | 5300 |
North America | 5000 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
continent
,sum(users) as users
from countries
group by continent
order by sum(users) desc;
END
movieDirectors
You want to expand your movie collection, but you don't really have any preferences so you're not sure where to start. After some consideration, you decide that you should start by finding more movies from award-winning directors whose movies you already own and who have shot a movie somewhat recently.
To find the directors whose movies you might want to consider watching in the first place, you've created a database of all the films you already own and stored them in a moviesInfo table, which has the following structure:
title
: the title of the movie;director
: the director of this movie;year
: the year the movie was released;oscars
: the number of the Academy Awards this movie received.
Given the moviesInfo table, compose the list of directors you should consider watching more movies from. The resulting table should have a single director
column and contain the names of film directors such that:
- they shot movies after the year
2000
; - the total number of Oscar awards these movies received is more than
2
.
The table should be sorted by the directors' names in ascending order.
Example
For the following table moviesInfo
title | director | year | oscars |
---|---|---|---|
BoBoiBoy: The Movie | Nizam Razak | 2016 | 0 |
Inception | Christopher Nolan | 2010 | 4 |
Interstellar | Christopher Nolan | 2014 | 1 |
Munna Bhai M.B.B.S. | Rajkumar Hirani | 2003 | 0 |
My Dear Brother | Ertem Egilmez | 1973 | 0 |
Rocky John | G. Avildsen | 1976 | 3 |
The Nights of Cabiria | Federico Fellini | 1957 | 1 |
The Sixth Sense | M. Night Shyamalan | 1999 | 6 |
The Sixth Sense | M. Night Shyamalan | 1999 | 6 |
Tokyo Story | YasujirĂ´ Ozu | 1953 | 0 |
Yojimbo | Akira Kurosawa | 1961 | 1 |
the output should be
director |
---|
Christopher Nolan |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select director
from moviesInfo
where year > 2000
group by director
having sum(oscars) > 2
order by director;
END
travelDiary
You are an avid traveler and you've visited so many countries that when people ask you where you've been, you can't even remember all of them! Luckily, every time you travel somewhere you write down the trip information in your diary. Now you want to get a list of all the different countries that you have visited using the information in your diary.
The diary is represented as a table diary, which has the following columns:
id
: the unique ID of the trip;travel_date
: the date the trip began;country
: the country to which you traveled.
Given this diary table, create a semicolon-separated list of all the distinct countries you've visited, sorted lexicographically, and put the list in a table that has a single countries
column.
Example
For the following table diary
id | travel_date | country |
---|---|---|
1 | 2008-05-12 | Ireland |
2 | 2010-11-04 | France |
3 | 2005-10-02 | Australia |
4 | 2008-06-08 | Japan |
5 | 2010-08-27 | Austria |
6 | 2009-02-15 | France |
the output should be
countries |
---|
Australia;Austria;France;Ireland;Japan |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select group_concat(distinct country order by country separator ';') as countries from diary;
END
soccerPlayers
You have a table soccer_team that contains information about the players in your favorite soccer team. This table has the following structure:
id
: the unique ID of the player;first_name
: the first name of the player;surname
: the last name of the player;player_number
: the number that the player wears (the number is guaranteed to be unique).
Create a semicolon-separated list of all the players, sorted by their numbers, and put this list in a table under a column called players
. The information about each player should have the following format: first_name surname #number
.
Example
For the following table soccer_team
id | first_name | surname | player_number |
---|---|---|---|
1 | Alexis | Sanchez | 7 |
2 | Petr | Cech | 33 |
3 | Hector | Bellerin | 24 |
4 | Olivier | Giroud | 12 |
5 | Theo | Walcott | 14 |
6 | Santi | Cazorla | 19 |
the output should be
players |
---|
Alexis Sanchez #7; Oliver Giroud #12; Theo Walcott #14; Santi Cazorla #19; Hector Bellerin #24; Petr Cech #33 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select group_concat(first_name, ' ', surname, ' \#', player_number order by player_number separator '; ') as players from soccer_team;
END
marketReport
Your company is planning to expand internationally very soon. You have been tasked with preparing a report on foreign markets and potential competitors.
After some investigation, you've created a database containing a foreignCompetitors table, which has the following structure:
competitor
: the name of the competitor;country
: the country in which the competitor is operating.
In your report, you need to include the number of competitors per country and an additional row at the bottom that contains a summary: ("Total:", total_number_of_competitors)
Given the foreignCompetitors table, compose the resulting table with two columns: country
and competitors
. The first column should contain the country name, and the second column should contain the number of competitors in this country. The table should be sorted by the country names in ascending order. In addition, it should have an extra row at the bottom with the summary, as described above.
Example
For the following table foreignCompetitors
competitor | country |
---|---|
Acme Corp | USA |
GLOBEX | USA |
Openmedia | France |
K-bam | USA |
Hatdrill | UK |
Hexgreen | Germany |
D-ranron | France |
Faxla | Spain |
the output should be
country | competitors |
---|---|
France | 2 |
Germany | 1 |
Spain | 1 |
UK | 1 |
USA | 3 |
Total: | 8 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
create temporary table t (country text, competitors int);
insert into t (select country, count(country) from foreignCompetitors group by country order by country);
insert into t (select 'Total:', count(*) from foreignCompetitors);
select * from t;
drop table t;
END
Specialties
interestClub
Implement the missing code, denoted by ellipses. You may not modify the pre-existing code.
You are the chairman of your university's drawing club, which isn't doing very well right now. The club meets two times a week to exchange drawing advice, talk about new techniques, and draw something together. But the members are starting to get bored during these meetings, so you've decided to add an additional activity to the routine.
In order to do this, you decided to collect information about the students, which is now stored in the table people_interests, which has the following columns:
name
- the unique name of a person;interests
- the set of interests or hobbies this person has, given as a comma-joined string. This column has datatypeset('reading','sports','swimming','drawing','writing','acting','cooking','dancing','fishkeeping','juggling','sculpting','videogaming')
.
This information gave you the idea that reading might be an interesting theme for the next meeting, so you announced that the next meeting will be reading-related. Now you're interested in the number of members that will come.
Given the people_interests table, find the people who will attend the next meeting, i.e. those who are fond of both drawing and reading. The resulting table should consist of a single name
column, and the records should be sorted by people's names.
Example
For given table people_interests
name | interests |
---|---|
August | cooking,juggling |
Buddy | reading,swimming,drawing,acting,dancing,videogaming |
David | juggling,sculpting |
Dennis | swimming,cooking,fishkeeping |
James | reading,drawing |
the output should be
name |
---|
Buddy |
James |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
SELECT name
FROM people_interests
WHERE interests & ... AND interests & ...
ORDER BY name
CREATE PROCEDURE solution()
SELECT name
FROM people_interests
WHERE interests & ... AND interests & ...
ORDER BY name
personalHobbies
You've been looking for new friends on the Internet. You just came across a cool website that helps people all over the world become friends by suggesting perfect friend candidates based on the information in users' profiles.
The system suggested some people for you to reach out to, and you're ready to make a move. However, you don't want to exercise your communication skills in vain, which is why prior to contacting a person you want to make sure you'll have something in common to talk about. The best option is to check whether they have the same hobbies you do, which are sports and reading.
You downloaded the list of suggested people and saved it in the people_hobbies table, which has the following structure:
name
- the unique person's name;hobbies
- a list of hobbies the person has (this column is of the datatypeset
).
Given the people_hobbies table, your goal is to return the sorted names of people who have sports and reading in their list of hobbies
.
Example
For the given table people_hobbies
name | hobbies |
---|---|
Adam | swimming |
Amy | reading,sports,swimming |
Carl | filmwatching,writing |
Carol | reading,swimming |
Deril | sports |
Jake | reading,sports,swimming |
Lola | reading,sports,painting |
Nina | sports,painting |
Sam | sports |
the output should be
name |
---|
Amy |
Lola |
As you can see, only Amy and Lola have both reading and sports in their hobbies
lists.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select name
from people_hobbies
where hobbies like '%sports%'
and hobbies like'%reading%';
END
booksCatalogs
You have your very own library at home, and it's getting bigger and bigger with each passing month. You've decided to create a database in which to store information about your books, in the hope that it will help you remember which books you have in your library.
Information about the books in your library is stored in the table catalogs, which contains the following columns:
doc_id
- the unique ID of the catalog;xml_doc
- the catalog as an XML file in the following format:<catalog> <book id="..."> <author>...</author> <title>...</title> </book> <book id="..."> <author>...</author> <title>...</title> </book> ... </catalog>.
Each catalog represents the work of one distinct <author>
in your library. There is exactly one <catalog>
element in each xml_doc
, and the id
for each book is unique.
Given the catalogs table, you want to find out which authors you have represented in your library. Your task is to create a new table with the author
column that will contain all the distinct authors, sorted by their names.
Example
For given table catalogs
doc_id | xml_doc |
---|---|
1 | <catalog> <book id="11"> <author>Chuck Palahniuk</author> <title>Fight Club</title> </book> <book id="12"> <author>Chuck Palahniuk</author> <title>Survivor</title> </book> </catalog> |
2 | <catalog> <book id="21"> <author>Bernard Werber</author> <title>Les Thanatonautes</title> </book> </catalog> |
3 | <catalog> <book id="31"> <author>Boris Vian</author> <title>The Big Sleep</title> </book> <book id="32"> <author>Boris Vian</author> <title>The Lady in the Lake</title> </book> <book id="33"> <author>Boris Vian</author> <title>The World of Null-A</title> </book> </catalog> |
the output should be
author |
---|
Bernard Werber |
Boris Vian |
Chuck Palahniuk |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
/*I got tired of trying to work out this out (picked at it for two months) and in true engineer's fassion, i'm stealing it from the internets... yay google*/
SELECT substring_index(ExtractValue(xml_doc, '//catalog//book//author'),' ', 2) as author
FROM catalogs
order by author;
END
habitatArea
As a young naturalist, you've been studying the inhabitants of the nearby woods for the past several months. You've just come across some footprints you've never seen before. To learn more about the habitat of the animal that left them, you marked the footprints locations on your map.
The information about the places where the animal left its footprints is stored in the table places. Here is its structure:
x
: thex
-coordinate of the place;y
: they
-coordinate of the place.
It is guaranteed that pairs (x, y)
are unique.
Now you want to find the area of the animal's habitat. You decided that the convex hull of the marked points is a good first approximation of the habitat, so you want to find the area of this hull.
Given the places table, write a select statement which returns only one column area
and consists of a single row: the area of the convex hull. It is guaranteed that the resulting area is greater than 0
.
Example
For the following table places
x | y |
---|---|
0 | 0 |
1 | 2 |
2 | 1 |
5 | 1 |
5 | 2 |
the output should be
area |
---|
6.5 |
Here is an illustration of the given points and their convex hull:
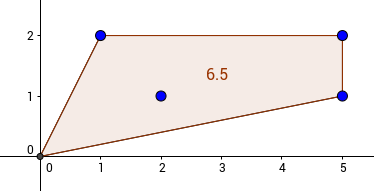
Note that you should return the exact answer without any trailing zeros.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
set @g = (select concat('MULTIPOINT(', GROUP_CONCAT(concat(x,' ',y) SEPARATOR ','),')') from places);
select ST_Area(ST_ConvexHull(ST_GeomFromText(@g))) as area;
END
WHEN was it the CASE
orderOfSuccession
The kingdom has been given terrible news: the King has passed away. While the nation is mourning, the noblemen need to decide who will take the throne next.
The late King had many children, and now it is necessary to determine their order of succession according to their seniority.
The list of the King's children is represented as a table Successors with the following attributes:
name
: the child's name;birthday
: the date of their birthday (it is guaranteed that birthday dates are unique);gender
: their gender (a character equal to'M'
or'F'
).
The resulting table should contain the names of the King's heirs in order of their succession to the throne as determined by their age, and preceded by their potential future titles (i.e. "King name"
or "Queen name"
).
Example
For the following table Successors
name | birthday | gender |
---|---|---|
Amelia | 1711-06-10 | F |
Anne | 1709-11-02 | F |
Caroline | 1713-06-10 | F |
Frederick | 1707-02-01 | M |
Loisa | 1724-12-18 | F |
Mary | 1723-03-05 | F |
William | 1721-04-26 | M |
The output should be
name |
---|
King Frederick |
Queen Anne |
Queen Amelia |
Queen Caroline |
King William |
Queen Mary |
Queen Loisa |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
case when gender = 'M'
then concat('King ', name)
else concat('Queen ', name)
end as name
from Successors
order by birthday asc;
END
orderingEmails
You've started to receive a lot of emails every day, and you decide to sort them in order to make it quicker to navigate through them.
Information about your emails is stored in a table emails, which has the following structure:
id
: the unique email id;email_title
: the title of the email;size
: the size of the email's body in bytes.
You decide to sort all the emails by their body sizes in descending order, because you think that the bigger an email is the more important it is. However, you don't like having the sizes written in bytes because they are usually too large and don't make much sense. You want them to be written in kilobytes (1 Kb = 210 bytes
) and megabytes (1 Mb = 220 bytes
) instead, rounded down if necessary. For example, 21432432
bytes is equal to 20
megabytes and 460912
bytes, so the result should be rounded down to 20 Mb
.
Given the table emails, build a table as follows: The resulting table should have the columns id
, email_title
, and short_size
, and should be sorted in descending order by the initial sizes of the emails. It is guaranteed that all the emails are of unique sizes, so there will not be any ties.
Example
For the following table emails
id | email_title | size |
---|---|---|
1 | You won 1M dollars! | 21432432 |
2 | You are fired | 312342 |
3 | Black Friday is coming | 323 |
4 | Spam email | 23532 |
5 | Your requested backup | 234234324 |
the output should be
id | email_title | short_size |
---|---|---|
5 | Your requested backup | 223 Mb |
1 | You won 1M dollars! | 20 Mb |
2 | You are fired | 305 Kb |
4 | Spam email | 22 Kb |
3 | Black Friday is coming | 0 Kb |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
id
,email_title
,case
when size < power(2, 20)
then concat(truncate(size / power(2,10), 0), ' Kb')
else concat(truncate(size / power(2,20), 0), ' Mb')
end as short_size
from emails
order by size desc;
END
placesOfInterest
You are trying to decide where you want to go on your vacation, so your travel agency has provided you with a database of possible destinations.
This database contains the table countryActivities, which has the following structure:
id
: the unique id of the record;country
: the country name;region
: the region of this country;leisure_activity_type
: the type of activity provided in the region. This can only be equal to one of the following values:Adventure park
,Golf
,Kart racing
,River cruise
;number_of_places
: the number of resorts in the region at which you can do this activity.
You want to see how many resorts in each country provide the activities you are interested in before you decide where to go on your vacation, but it's hard to do this using only the table provided by your travel agency. To make things easier, you have decided to create a new table with a better structure.
Given the countryActivities table, compose the resulting table with five columns: country
, adventure_park
, golf
, river_cruise
and kart_racing
. The first column should contain the country name, while the second, third, fourth, and fifth columns should contain the number of resorts in the country that offer Adventure park
, Golf
, River cruise
, and Kart racing
, respectively. The table should be sorted by the country names in ascending order.
Example
For the following table countryActivities
id | country | region | leisure_activity_type | number_of_places |
---|---|---|---|---|
1 | France | Normandy | River cruise | 2 |
2 | Germany | Bavaria | Golf | 5 |
3 | Germany | Berlin | Adventure park | 2 |
4 | France | Ile-de-France | River cruise | 1 |
5 | Sweden | Stockholm | River cruise | 3 |
6 | France | Normandy | Kart racing | 4 |
the output should be
country | adventure_park | golf | river_cruise | kart_racing |
---|---|---|---|---|
France | 0 | 0 | 3 | 4 |
Germany | 2 | 5 | 0 | 0 |
Sweden | 0 | 0 | 3 | 0 |
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
select
country
,sum(case when leisure_activity_type = 'Adventure park' then number_of_places else 0 end) as adventure_park
,sum(case when leisure_activity_type = 'Golf' then number_of_places else 0 end) as golf
,sum(case when leisure_activity_type = 'River cruise' then number_of_places else 0 end) as river_cruise
,sum(case when leisure_activity_type = 'Kart racing' then number_of_places else 0 end) as kart_racing
from countryActivities
group by country
order by country;
END
soccerGameSeries
You have a table scores that contains information about a series of soccer games. Your goal is to determine the winner of the series. A team is declared the winner if it won more games than the other team. If both teams had the same number of wins, then the winner is determined by the better goal difference (the difference between the goals that a team scores and the goals that the opposing team scores on them over all the games). If the goal differences are equal, the winner is the team that scored more goals during away games (i.e. games when it was not the host team). The result is the index of the winning team. If the above criteria are not sufficient for determining the winner, return 0
.
The scores table contains the following columns:
match_id
- the unique ID of the match;first_team_score
- the score of the1st
team in the current match;second_team_score
- the score of the2nd
team in the current match;match_host
- the team that is the host of the match (can be1
or2
).
Your task is to write a select statement which returns a single column winner
, which can contain 1
, 2
, or 0
.
Example
For given table scores
match_id | first_team_score | second_team_score | match_host |
---|---|---|---|
1 | 3 | 2 | 1 |
2 | 2 | 1 | 2 |
3 | 1 | 2 | 1 |
4 | 2 | 1 | 2 |
the output should be
winner |
---|
1 |
The first team won 3
games out of 4
, so it's the winner of the series.
- [execution time limit] 10 seconds (mysql)
CREATE PROCEDURE solution()
BEGIN
set @first_team_wins = (select count(*) from scores where first_team_score > second_team_score);
set @second_team_wins = (select count(*) from scores where first_team_score < second_team_score);
set @first_team_total_score = (select sum(first_team_score) from scores);
set @second_team_total_score = (select sum(second_team_score) from scores);
set @first_team_away_score = (select sum(first_team_score) from scores where match_host = 2);
set @second_team_away_score = (select sum(second_team_score) from scores where match_host = 1);
select case
when @first_team_wins > @second_team_wins then 1
when @first_team_wins < @second_team_wins then 2
else case
when @first_team_total_score > @second_team_total_score then 1
when @first_team_total_score < @second_team_total_score then 2
else case
when @first_team_away_score > @second_team_away_score then 1
when @first_team_away_score < @second_team_away_score then 2
else 0
end
end
end as winner;
END